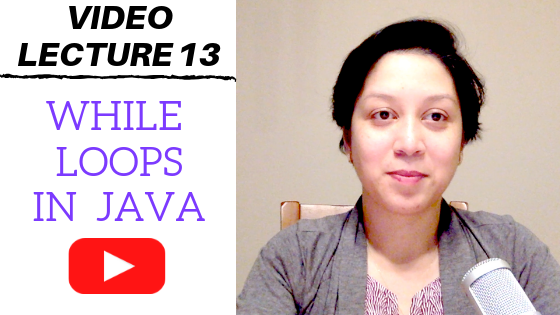
While loops in Java: Video Lecture 13
Java has three types of loops: (1) for loop, (2) while loop, and (3) do-while loop. This post focuses on while loops in Java. In the video-lecture associated with this post, we compare side-by-side for loops and while loops.
Contents
While loops in Java
The while loop in Java has the following structure.
while (condition){
//Code block
}
The variable condition
must be a result of a boolean expression. That is, the variable condition
is a boolean value (true
/false
). When the variable condition
is true
, the execution goes inside the code block. After executing the code block, the virtual machine (VM) comes back to the while condition
to check if it is still true. If VM finds that the variable condition
is still true, the execution goes inside the code block again.
VM keeps repeating the code block as long as the variable condition
is true
. If in any execution of the code block the value of the variable condition
becomes false, then the VM does not go inside the next while-check. Hence the loop stops executing.
Problem Used in the Video
Print all the numbers between 1 and 10, separated by spaces. That is, the terminal output should look like the following one.
1 2 3 4 5 6 7 8 9 10
Of course, we are not going to hardcode ten numbers in a System.out.print statement. We will use a loop to print all the ten numbers. In the video, we will first use a for loop to print the ten numbers. Then, we will modify the for loop to a while loop. The output of the program will remain the same after the changes in the code.
The Solution
The solution using a for loop is provided below.
class WhileTest{
public static void main(String[] args){
int i;
for (i=1;i<=10;i=i+1){
System.out.print(i+" ");
}
}
}
The solution using a while loop is provided below.
class WhileTest{
public static void main(String[] args){
int i;
i=1;
while(i<=10){
System.out.print(i+" ");
i=i+1;
}
}
}
The Video Lecture
Here is the video lecture.
Transcription of the Audio of the Video
We are going to discuss another looping technique known as While-Loop. Everything we did using Java for-loops in the previous video lectures can be done using while-loops. Watch the video till the end for a detailed explanation on while-loops, a comparison between Java for-loops and while-loops, and a coding-example to explain how while-loops work.
While loop in Java
Hi, I am Dr. Monika Akbar. I am explaining Java while-loops today. A Java while-loop has this structure.
// Hand-draw
while (condition){
// The code-block under the scope
}
In the while-loop structure, you have a “condition” to control the repetition of the loop. As long as this condition is true, the execution will keep going inside the while-loop and run the code-block again and again.
Inside the code-block, you can write something that will make the condition false after some number of iterations.
For loop in Java
Let us compare the while-loop structure with a for-loop structure. Let us quickly write the for-loop structure.
//Hand-draw
for (initialization; condition; change){
// The code block under the scope
}
We have initialization, condition, and a change to control the for-loop. In the while-loop, we are missing the initialization, and the change. We have the condition part only. In an ideal looping, along-with the basic while-loop structure, we will need to include initialization and the change part, this way.
Initialization and change in a while loop
// Hand-draw
initialization;
while (condition){
// The code block under the scope
change;
}
Let us discuss this using an easy-to-solve example problem. Let us assume that the problem is to print all the numbers between 1 and 10 separated by spaces.
We are starting with a for loop
We can quickly write a for-loop, in which we use an integer variable i to control the loop. In the initialization, i is equal to 1. The condition is that i is smaller than or equal to 10. That means, as long as, the variable i remains within the range of 1 to 10, the loop will keep repeating. At the end of each iteration, i will increment by 1, as stated by the third part of the for-syntax.
We print the variable i inside the code. We put a space after i, so that on the terminal a gap is created between the current value of i and the next one.
Please save the file, compile it, and run the generated class file. All the integer numbers from 1 to 10 are printed on the terminal.
To make sure that there is no confusion, this part, containing “My Computer” is my command prompt. The text “My Computer” is not an outcome of the program we have written.
Now, we will use a while-loop instead of the for-loop to do the same task, that is printing the numbers from 1 to 10.
Difference between a for loop and a while loop in Java
Notice that there are some structural differences between a for loop and a while-loop. The basic while-loop only has the “condition” part for which the iteration will go inside. We will include the initialization part outside the while-loop, and the “change” part inside the code-block of while-loop.
Using while loop in Java
Let us keep the line that contains System.out.print and delete the for structure.
Let us write the while statement. In parentheses we will write the condition for which the System.out.print statement will be executed. If the condition is true, everything inside the curly brackets will be executed.
For the initialization of i, let us write i=1 before the while statement.
After printing the current value of i, we do not want i to retain the same value because we want to print the next number in the next iteration. Therefore, we will change i to the next number. To do that we write i=i+1; right after where the current value of i is printed using the System.out.print statement.
Note that after this code segment executes, a number is printed and the value of i becomes one more than the number that was printed.
This line i=i+1 is responsible for the increment of i. Now, notice that the initialization is i=1. The loop will iterate and at the end of the iteration, the value of i will increase. We need to write a condition within the parentheses of the while-statement. The condition should be such that, the execution goes inside when the value of i is smaller than or equal to 10.
The condition is exactly the same one we had in the for-loop.
The output of the new program
This newly written code is an alternative of the code we wrote using a for-loop. The output of the code should be the same as before.
Let us save the file, compile the code, and execute the generated class file. Notice that we have exactly the same output, where we have all the 10 numbers starting from 1 and ending with 10. The numbers are separated by spaces.
Let us do a side by side comparison of the for-loop and the while-loop. The initialization of the for loop is a part of the structure of the for loop. In the while-loop, we wrote an initialization of the control-variable outside the while loop.
The condition is a part of the for-loop. The condition is a part of the basic while-loop too.
The increment operation is a part of the for-structure. The increment operation was not a part of the basic while-structure. However, we can incorporate the increment, or the change operation inside the code-block of the while loop.
In reality, the for-loop and the while loop can do the same thing.
Concluding remarks
We already learned nested for-loops in the previous video. Similar to nested for-loops, you can write nested while loops. You can even write one while-loop inside a for-loop, or even one for-loop inside a while-loop. The summary is, you can replace a for-loop by a while-loop and vice versa.
I hope the concept of while loop is clear to you. Please let us know if you have any question through the comment section below. If you haven’t subscribed to our website Computing4All.com and to the YouTube channel where this video is hosted, please do so to receive notifications on our new articles and video lectures. We wish you become successful in learning to code. Again, please do not hesitate to contact us via the comments section below, if you have any question. Or, even to introduce yourself. See you soon in another video.