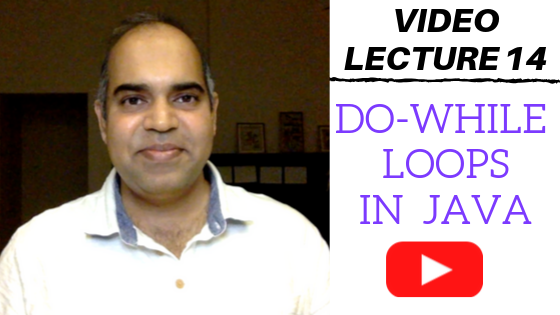
Java do-while Loop: Video Lecture 14
Java do-while loop is one of the three looping techniques available with the language. The other two loops are: for loop and while loop. do-while is a little bit different than for or while loops. In the “for” or “while” loop, generally, a condition is checked in the beginning to realize whether the execution should go inside the loop, or not. In contrast, do-while checks for the requirement of iteration at the end of the loop. As a result. do-while would execute the code-block inside the loop at least once.
Contents
- 1 Java do-while Loop
- 2 Problem Used in the Video
- 3 The Solution
- 4 The Video Lecture on Java do-while Loop
- 5 Transcription of the Audio of the Video
- 5.1 Why do you need a do-while loop?
- 5.2 The structure of Java do-while loop and how it compares with other loops
- 5.3 What is a common use of Java do-while loop
- 5.4 Starting the code
- 5.5 Scanner
- 5.6 Java do-while loop
- 5.7 A character variable
- 5.8 Get user-intention regarding repetition
- 5.9 Single quotes for a character
- 5.10 The goodbye message
- 5.11 Save, compile, and run
- 5.12 Concluding remark
- 6 Subscribe
Java do-while Loop
Java do-while loop has the following structure.
do { // Code-block } while (condition);
The condition is generally an expression that results in either true or false. If the condition results in true, another iteration of the code-block will occur. If the condition becomes false, the loop terminates and executes anything found after the while loop.
Problem Used in the Video
In the video, we demonstrate how to write a program using java do-while loop to repeat the same task based on user preference. The task that the program repeats is the addition of two number provided by the user. The following image provides a screenshot of the program.
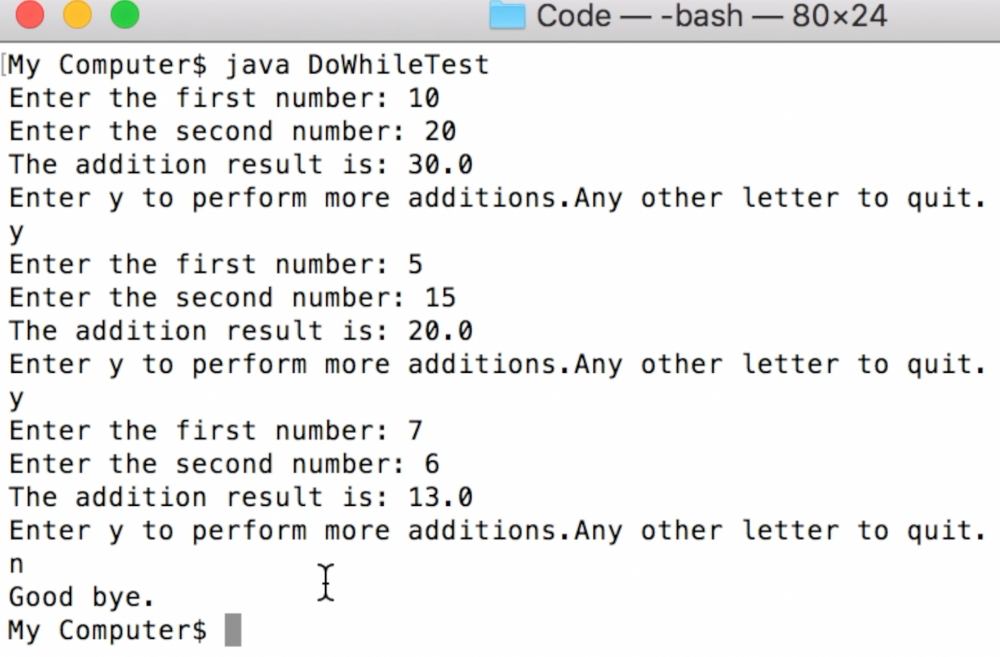
Notice that the program asks for two numbers from the user. The program prints the summation of the two numbers. Then it gives the user to enter ‘y’ to continue and any other letter to quit the program. The user repeats the task several times by entering ‘y’. When the user enters ‘n’ to quit, the program outputs “Good bye.” and terminates.
We wrote the program using a Java do-while loop. Here is the solution.
The Solution
The solution is provided in the space below.
import java.util.Scanner;
class DoWhileTest{
public static void main(String[] args){
double a;
double b;
Scanner scan = new Scanner(System.in);
char decision;
String str;
do{
System.out.print("Enter the first number: ");
a=scan.nextDouble();
System.out.print("Enter the second number: ");
b=scan.nextDouble();
System.out.println("The addition result is: "+(a+b));
System.out.print("Enter y to perform more additions.");
System.out.println("Any other letter to quit.");
str=scan.next();
decision = str.charAt(0);
}while (decision=='y');
System.out.println("Good bye.");
}
}
The Video Lecture on Java do-while Loop
The video lecture below explains a lot of details on Java do-while loop.
Transcription of the Audio of the Video
In this video, we are going to discuss another looping technique known as do-while loops. We will discuss the structure of the do-while loop and compare it with other looping techniques in Java. Definitely, we will write a program to explain how a do-while loop works.
Why do you need a do-while loop?
Hi, I am Dr. Shahriar Hossain. Today, I am going to explain do-while loops in Java. From the previous videos, we are already familiar with for-loops and while-loops. Now the question is, why do we need a third type of loop, named do-while? Its a Good question. A do-while loop is a special kind of looping technique that is suitable for a situation when execution of a code-block is required at least for once.
Now, you may ask if a programmer needs to absolutely know about do-while loops. The answer is, a programmer can avoid do-while loops and use for or while loops with some tricks to do the same work. However, knowing do-while loops makes a programmer’s life easier in some situations.
Moreover, if you have to sit for a programming exam, or go for an interview, you absolutely need to be familiar with do-while loops. Do-while is one of the three looping techniques. As a programmer, we should be familiar with all the three type of loops: for, while, and do-while.
The structure of Java do-while loop and how it compares with other loops
Anyway, let us take a quick look at the structure of a do-while loop.
// Show Handwritten animation
do {
// code-block
} while (condition);
-----
while (condition){
// code-block
}
-----
for (initialization; condition; change){
// code-block
}
The do-while loop starts with the word do. Then the scope of the code-block starts with a curly bracket. At the end of the code block, you end the scope using a closing curly bracket. Then the syntax “while” is written at the end with a condition. We have to put a semicolon at the end of the do-while loop.
Notice that do-while is quite different than while loop and for loop. Do-while is different in the sense that in while and in for loops, the condition is executed before going inside the scope. If the condition results in “true” in a for or while loop, the execution goes inside. If the condition results in false, the condition does not go inside.
In contrast, there is no condition before going inside the code-block of the do-while loop. That means the code-block will execute at least once. At the end, you have the condition with the while. Every time, the code block is executed, the condition-check will evaluate whether another repetition of the code-block is required. If the condition is true, then the code-block will be executed again. Otherwise, the execution will move forward to whatever statements are there outside the do-while loop.
What is a common use of Java do-while loop
A common use of do-while is to check if the user wants to repeat the same work. Let us discuss a program that uses do-while to repeat the same work as long as the user wants it to repeat.
<<Show the terminal output of the program first>>
Consider that we have an amazing program that can add two double-precision numbers. The program asks the user for two numbers. The program then reports the addition result. After the addition, the program asks the user if she/he would like to add more numbers. If the user enters ‘y’ for yes, then the program will ask again for two numbers that the user wants to add.
After the user enters two new numbers, the program will report the new addition result, and ask if the user wants to do more additions. The process will keep repeating as long as the user enters ‘y’. If the user enters ‘n’, then the program will say “Good bye” and terminate.
Starting the code
Let us write code for this program.
At first let us write a program that asks for two numbers, reports the summation result, and then terminates. Later we will modify the code to repeat the task.
Scanner
We already know how to write a Scanner object and ask the user to enter two numbers. We keep the two numbers in two variables named a and b. After receiving the numbers from the user, all we have to do is, to print the summation of the two numbers.
If we compile the java file and run the generated class file, we will notice that the program asks for two numbers from the user, then the program reports the summation, and finally the program terminates.
Java do-while loop
Now, what we want to do is the following – we want to use a do-while loop to repeat this part of the code, where we ask the user for two numbers, and then print the summation result.
Let us write the “do” syntax, and put our code inside the scope of the loop. The loop ends with the syntax “while” and then in parentheses we will need to provide the condition that is responsible for the repeat. Note that we have to put a semicolon at the end of the do-while loop.
Now, we still do not have any information that we can use for the condition that should go here. In fact, with the current code-block, we did not collect any information from the user regarding her or his desire to repeat the addition for another set of numbers.
A character variable
Let us declare a character variable that will help us to store a letter representing user’s desire to repeat. A character variable is able to keep only a letter, digit, or a symbol in it. For example, at can hold, ‘a’, ‘b’, ‘c’, or any letter. A character can hold even a digit like ‘1’ or ‘2’, or ‘3’. It can even hold symbols like ‘+’, ‘-’, or ‘*’. There is a wide range of characters, letters, digits, or symbols that it can hold.
We will ask the user to enter ‘y’ if she or he wants to do another addition. We will ask the user to enter anything else if she or he does not want to do any more addition. We will store whatever the user entered in the character variable named decision, we just created.
Get user-intention regarding repetition
We use the scanner object to get the user input for a character value. Unfortunately, the scanner object does not have a direct method to read a character. We have to read whatever the user enters using a the next() method. We have to keep the information in a String object. str is the String variable. Then we have to retrieve whatever we have in the first position of the String str and put it in the character variable decision. The value zero in the parentheses of charAt gives the first character. Characters in a String starts from 0. That is, the first character of a String has a location of 0, the second character has a location 1, the third character has a location of 2, so and so forth.
Anyway, we just need to take a look at the first character, whether it is ‘y’ or not. If the first character is ‘y’, it will be in location 0 of the String str. Hence, the variable decision will contain ‘y’.
If we find that the variable decision is not ‘, then we do not have repeat anymore.
Now, notice that the repetition of this do-while is dependent on whether the variable decision is ‘y’ or not. Therefore, we can write the condition here as decision ==‘y’. That means, if the decision variable is the character ‘y’, then repeat, otherwise do not repeat.
Single quotes for a character
Note that a character is placed within a single start quote and a single end quote. Without the quotes, the compiler will think that ‘y’ is a variable and will throw and error stating that the variable y is not declared in this program. Therefore, to indicate that we are referring to the exact character value ‘y’, we have to provide the single quotes.
The goodbye message
After the do-while loop terminates, we want the program to write a “Good bye” message. Therefore, we write a System.out.printlin method outside of the do-while loop.
Save, compile, and run
Let us save the file, compile the code, and execute the generated class file. The user is asked for two numbers. The user enters the two numbers. The program reports the addition result. Then the program asks to enter y, if the user wants to do more additions. The user types ‘y’ and hits the enter button. The program asks for the numbers again. The addition result is displayed. Then the program again asks whether the user wants to do more additions. The user can keep entering ‘y’ here and keep doing additions. When the user enters anything other than ‘y’, the program says “Good bye” and then terminates.
Notice again, how we wrote our program. The code block executes at least once. The decision whether to repeat the code-block or not is made at the end. This structure of do-while is different than for loop and while loop in this way.
Concluding remark
Through this video, we have covered the third looping technique used in Java. We already learned nested for-loops one of the previous videos. Similar to nested for-loops, you can write nested do-while loops. You can even write one do-while-loop inside a for-loop, or even one for-loop inside a do-while-loop. I hope the concept of looping is clear to you. Please let us know if you have any question through the comment section below. If you haven’t subscribed to our website Computing4All.com and to the YouTube channel where this video is hosted, please do so to receive notifications on our new articles and video lectures.
We wish you become successful in learning to code. Again, please do not hesitate to contact us via the comments section below, if you have any question. Or, even to introduce yourself. See you soon in another video.
8 Comments
Thank you so much. I appreciate your quick response and the explanation.
You are welcome. We wish you a wonderful new year.
str = scan.next();
decision = str.charAt(0); I really did not understand this area well. why is the scan.next without String or any other variables? then the charAt.
Thank you for the question.
next() does not have any parameters. This is how the method is written in the Scanner class. Notice that reading the next entry really does not require input from the caller.
charAt has a parameter. This is how it is written in the String class. Notice that, we need the parameter because we have to tell the method which character we want to retrieve from the string.
We have covered methods and parameters in a later video, which might clarify how methods are written: https://computing4all.com/java/methods-in-java-programming-language/
Please note again that we have not yet covered classes and objects, and hence it will not make much sense to describe Scanner and String from class/object perspective.
Thanks again.
I am following up. It is really simplifying. I would want some direction on how to turn this code into a read time project.
The following link has some indication on how to get the System time: https://stackabuse.com/how-to-get-current-date-and-time-in-java/. Since we have not yet taught classes and objects, it will be hard to explain this. Thank you for your question.
Good afternoon. My name is Richard. Am writing from Ghana. I say thank you for providing this wonderful channel . your tutorials are one of the best and has helped to understand basic java. Continue the good work.
Dear Richard,
Greetings from Texas, USA. I appreciate your feedback and your comment. We will keep posting the video lectures. I hope the lectures will help learners all over the world.
Please do not hesitate to contact us if you have any question regarding any of the materials.
Thank you!
Best regards,
Shahriar