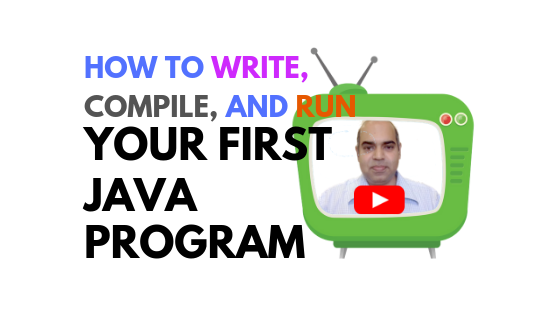
Writing Your First Java Program: Video Lecture 1
Writing the first program in Java can be intimidating, especially if someone is learning a programming language for the first time. Please do not be afraid or discouraged. If you saw someone write programs in the speed of light — especially in movies when there are only ten seconds left to a terrible happening, which only a nerdy programmer can stop — you have to understand that it is just a freaking movie.
In the following video, you will watch how you can write a program to display a sentence or two on the computer screen. Trust me, if you and I cannot write and generate the program to display a sentence in two minutes, the nerds in the movies will never be able to write a program to stop a terrible happening — for sure not in ten seconds. A big BOO to all those movies that create misconceptions regarding programming, computing, Artificial Intelligence, and Data Science.
Contents
- 1 The Video: How to Write, Compile, and Run Your First Java Program
- 2 Exercises
- 3 Comment
- 4 Transcription (of the audio of the video)
- 4.1 What you need for the exercise in the video
- 4.2 Editor
- 4.3 Starting of a Java code
- 4.4 Scope of a Java class
- 4.5 The Main method in a Java program
- 4.6 Writing an instruction
- 4.7 Compiling a Java code
- 4.8 Using the Terminal or a Command Prompt
- 4.9 Some commands like ls or dir, and clear or cls
- 4.10 Sequence: Write Code → Compile → Run
- 4.11 The javac command
- 4.12 The java command
- 4.13 Can we make the output esthetically better
- 4.14 How can we print a second sentence
- 4.15 Conclusion of the video
The Video: How to Write, Compile, and Run Your First Java Program
We created the video keeping new learners in mind. As a result, the video contains many details. We have plans to publish more videos on programming using Java in a sequence that we use to teach in a bachelors-level programming course. All the videos will explain topics keeping new learners in focus.
Exercises
If you want to practice more after watching the video, here are some follow-up exercises for you.
Write a Java program that displays the following texts on the terminal/command prompt.
Hello, world!!!
Java is cool!
I love mocha too.
I do not know why a programming language is named after coffee.
Write a Java program to display the following sentences on the terminal/command prompt.
I have 2 pencils on my desk and 3 in my drawer.
I have a total of 5 pencils.
Comment
I am pretty sure you will be able to modify the code we discussed in the video to solve the exercise above. We are planning to come up with the next video in this lecture series in a few days. To stay in touch, please subscribe to Computing4All.com. Enjoy!
Transcription (of the audio of the video)
Hi,
I am Dr. Shahriar Hossain. I am here today to help you with your first Java program.
The video is intended for people who have planned to start or have started recently to learn the Java programming language.
What you need for the exercise in the video
You will need a laptop or a desktop computer to practice the items I cover today. You will need three items, on your laptop or desktop, to practice the content I will cover today.
First, you will need a simple Editor. I will explain more about simple editors soon in this video.
Second, you will need the Java Development Kit SE installed on your computer. On Google, please search with the phrase Java Development Kit SE and you will get the link for a download. Install the Java Development Kit on your machine, if it is not already installed. (The link is here: Yes, you will need to download Java. You can download Java Development Kit SE from this link: https://www.oracle.com/technetwork/java/javase/downloads/index.html . One issue with direct link is that it may expire. This is why it is better to search using Google and then download the latest version.)
Third, you will need to use the terminal, which is already installed on your computer. On a Windows computer, it is called a Windows terminal, and on Mac, it is a Mac terminal.
Please keep watching the video, I will explain more when time comes.
I will try to explain everything slowly with details. Many times, we think about the coverage of materials and teach too fast in a university classroom. As a result, students sometimes struggle with the topics we cover. The struggle is not because the students do not have the background but many times it is because we, the professors, do not give students enough time to follow through.
This particular video is not from my classroom. A great thing about a video is that you can pause it and repeat as many times as you want. I am hoping that today’s material will give you a clear idea of how to write and run your first Java program.
In a few seconds, I will go to my computer and share the screen with you and demonstrate how to write a Java program, compile it, and execute the program YOU have written. I hope you will enjoy it.
Editor
I am going to write my first program. The name of the program is Welcome. This is why I named the program file that I am writing Welcome.java. I am writing Welcome.java using a plain text editor. A plain text editor is something like notepad on Windows. You cannot draw anything as you do on MS Word using a simple text editor. On a mac, you can use Xcode just like I am using it to write my program. Please note that you cannot use sophisticated document editors like MS Word to write your programs. Your program has to be written using a text editor. If you are using Windows, notepad is an excellent editor to start with. For Mac, Xcode is a good start.
Again, the name of the file on which I am writing now is Welcome.java.
Starting of a Java code
A Java code starts with the syntax class. Later, we will learn that there can be some other items even before writing the syntax “class”. For now, let us say that a java file starts with the word/syntax class. Then you have to write the class name. The class name is exactly the same as the name of the file but without the extension .java. Therefore, the name of the class is Welcome. Just welcome.
Scope of a Java class
After writing “class Welcome”, we have to tell the scope of the program. In Java, mentioning the scope of something is done using curly braces. I am typing an opening curly bracket here and a closing curly bracket here. Everything I will write for the program will be inside these curly braces. These curly braces are defining the scope of the program I am writing.
The Main method in a Java program
A java program starts with the main method. Later in another video in this sequence, I will explain what methods are. For now, we just need to know that a Java program starts from the main method. Inside the class, I am going to writing the main method. The syntax for the main method is “public static void main”. I will explain the meaning of each of these words in a future video.
Then in starting and closing parentheses write String start and close brackets args. This line is something that has a lot of unknowns at this point. The topics associated with each of the words in this line are covered a little later in a programming course. So, bare with me regarding this line. Just know that this line is a marker for the computer from where your program will start. We need a set of curly starting and ending curly braces to state the scope of the main method we are going to write. Whatever instructions we will write inside this scope of the main method, the computer will execute it.
Writing an instruction
Now, we will write our first instruction. A common standard in Java or in most of the programming languages is that one instruction should be written in one line. Later, we will see that one line may have multiple instructions too.
One question you may ask is, what instruction do we want to write. At this point assume that the purpose of the program we are writing is to display several sentences on the screen. Therefore, the instructions we will write are relevant to writing something on the screen.
The instruction, which is practically a Java method, is System dot out dot print. Then within parentheses write whatever you want to print. In this case, I am telling the computer to print “Welcome to the world of Java ” Whatever I want to print must be inside quotations. These are double quotations. At the end of each instruction, we must write a semicolon. The semicolon indicates the end of the instruction. Without the semicolon the computer will be lost regarding the end of the instruction.
Please make sure that you have this piece of code written before we go to the next step. The next step is to compile this code and generate the program. Then we will run the generated program to check if our program prints the sentence “Welcome to the world of Java ” on the screen.
Compiling a Java code
Again, I have written the code in a file named Welcome.java. The computer cannot directly execute a high-level program written by humans. We will use the Java compiler to generate a program, which is a file with .class extension. The computer can execute the class file.
When I said the computer can execute the class file, I meant that the Java virtual machine can execute the class file. Java virtual machine, which can execute our programs, is also called in its short name JVM.
Let me show you the entire process.
Using the Terminal or a Command Prompt
You have your code written in a file Welcome.java. You need to open the terminal now where you will be able to compile and execute the program you have written. The terminal on a Mac is called TERMINAL. On a Windows system, the Windows terminal is a program called CMD.exe. I am showing this example using my Mac terminal. The java commands are the same for all types of desktop and laptop computers.
A terminal is a program from which we can execute other programs. We will execute the Java Virtual Machine, as well as the Java Compiler from the terminal.
Notice that I am first typing the word java and pressing the enter button to check is java command is present on my computer. The command is showing me java-related parameters indicating that java is present on my computer. Then I am typing javac and pressing the enter button, which shows me some massages printed by the javac program. This is an indication that javac is already present in my computer. Therefore, I am ready to compile and run my program Welcome.java.
If your terminal displays a message saying “Command not found” when you type java or javac and hit the enter or return button, then your computer does not have java or the terminal is not recognizing the path that contains java virtual machine. Please make sure that you have installed java and your terminal recognizes the path of the Java Virtual Machine.
Some commands like ls or dir, and clear or cls
Then, I clear the terminal with the command clear. Now, I have to make sure that my terminal’s command prompt goes to the directory where I have Welcome.java. I use the command cd to reach to the folder where I have the file Welcome.java. To make sure that my command prompt is currently located in the directory where I have welcome.java, I just type ls, which shows me the content of the current folder. I see that the file Welcome.java is in the current directory. Therefore, I can execute the java compiler to generate the class file.
Sequence: Write Code → Compile → Run
I should mention at this point that the sequence of creating the program is the following: At first, write your code in a java file. Then compile the java file using javac, which is the java compiler. After compilation, a class file is generated. The class file is the program that Java virtual machine recognizes. Run the class file using the program java.
The javac command
So, I am now going to use javac to compile my Welcome program.
I typed javac Welcome and then hit the enter button. It gives me an error because I missed the .java extension. Then, I typed the javac command with Welcome.java, the full file name. Notice that a class file is generated in the folder immediately. The name of the class file is Welcome.class. Welcome.class is the actual program that java recognizes. Now, we will run the class Welcome.
The java command
To run the generated Welcome.class, we have to run the command java Welcome. The java does not require the .class extension in the command. Therefore, running the program is plain and simply java Welcome.
Notice that we wanted to print Welcome to the world of Java. The program has printed it on the terminal screen. If you have come up to this point of the video and practiced it on your computer, Many many congratulations on the execution of your first Java program.
Can we make the output esthetically better
Now, although the line is printed on the terminal, the command prompt appears right after the sentence ends, which is practically an eyesore. I would like to see the command prompt in a new line. The way how we solve the problem is, use System.out.println instead of System.out.print. Let us change the code and see if we can make the output esthetically better.
Now, that I have changed System.out.print to System.out.println, there should be a new line at the end of the displayed output on the terminal. We will have to compile the program using javac again because we have changed the code. To run the newly generated class file we again use the command java Welcome.
Notice that the output now contains “Welcome to the world of java …”, and the command prompt has been moved to the next line.
How can we print a second sentence
Now, suppose we want our program to display a second line containing “I love to code using Java.”
All we have to do is write another System.out.println instruction in our code. The parentheses of the System.out.println method will now contain “I love to code using Java.” After including the line, save the file Welcome.java.
Compile the code using javac because Welcome.java has been modified. Now run the class file using the java command. You have two lines as the output of the program. The first line contains “Welcome to the world of Java” and the second line contains “I love to code using Java.”
These two lines are printed on the terminal because using your code you told the computer to print them on the screen.
Now, you can print as many lines as you want on the terminal by writing as many System.out.println instructions as you need. In the future, we will learn more basic items of programming and move forward to solve complex problems.
Conclusion of the video
I hope that you enjoyed the video. I always tell my early stage classes in Computer Science that learning to program is not the hard part. The hardest part is to develop the skill through which you will solve problems. Practice makes women and men great in programming and problem-solving. Hope to see you soon with another video.
10 Comments
Dear Mr. Hossain,
There are various Java JDK SE from 7 – 14.
Please kindly advise which of the above is best I could download and install.
This is interesting and I need to learn more.
Awaiting your respond soon.
Please use Java SE Development Kit 14. I recommend JDK SE 14 because it is the latest one as of today. Thank you for your question.
Plz run your code on Windows operating system. I am not understand it correctly
Thank you for your message. Yes, we use UNIX-based commands, such as commands in Linux and MacOS. Regarding Java, Windows-based commands are the same. For the compiler, the command is still javac and for the virtual machine, the command is java.
However, making the commands available to the command prompt of Windows might be a hassle. It involves the system’s path variable.
For Windows installation of an editor and Java, you can use this link: https://introcs.cs.princeton.edu/java/windows/manual.php Please use the latest version of Java if the link states an older version.
For learning purpose, you can use an online Java compiler, which does not require installation of anything on your computer, like this one: https://www.onlinegdb.com/online_java_compiler. However, we do not recommend the use of online compilers for serious practice. After all, the target is to make sure that your program runs on any computer, not just on an online platform.
Thank you once again for your message. Please do not hesitate to let us know if you have any questions.
I have the app installed but needed something to push me. I think these videos will be give me that. Many thanks
Haha. We will keep posting new videos on Java programming if the videos help. Thank you for the comment.
Thank a lot for video.
We are glad to know that you liked the video. Thank you for watching and commenting.
very effective and inpirative video
We are glad to know that you liked the video. Thank you for your comment.