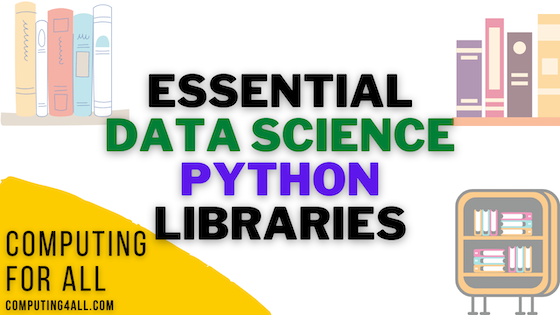
Essential Python libraries for data science
In recent years, there has been an explosion of Python library release for data science. Data scientists extensively use these libraries for applications in scientific domains, finance, economics, business, intelligence analysis, and many other fields. The libraries (also called packages) provide a comprehensive set of problem-solving algorithmic tools covering optimization, artificial intelligence, data mining, decision trees, and many more. From basic data engineering to analysis of refined information to forecasting to fine visualization to aid decision-making — you name it, everything is out there in Python.
I should also mention that these data science Python libraries are not like Aladdin’s magic lamp to solve all our problems. Python programming is a convenient tool — what we want to build is up to us. We still need a strong and creative mind to build models to retrieve meaningful information. A clear understanding of the algorithms and models is essential for every data scientist, whether they use Python or Matlab or R or Java.
In this article, I outline the data science Python libraries that I think are essential to remain equipped with what is out there in the data world. Of course, I am outlining the most important ones that are widely used. There are specialized ones that you might need to learn on the fly based on project requirements. There is no arguing that the more we know, the better we are prepared for our jobs in the industry or academia, where we face data-related problems daily.
Here are the most essential Python libraries for data mining, machine learning, and in general — data science.
Contents
Numpy
Numpy is a Python library with extensive functionality for operations over matrices and multidimensional arrays. It is common to transfer raw data to a tabular form because many algorithmic tools directly use tabular data. Tabular data is generally kept in the form of a matrix in a computer’s memory. Since Numpy includes essential matrix operations and mathematical functions to operate over the multidimensional arrays, data scientists use it almost every day to deal with tabular data. (Every day might be a weak term in the context of Numpy. I was just hesitant to say every hour.)
Numpy provides a flexible data structure for big data. One can combine multiple data structures and create new ones using Numpy. Many other libraries use Numpy due to its flexibility and availability of a rich set of functionality. Therefore, a data scientist using Python programming cannot work much without knowing the basic functionality of Numpy.
Pandas
The Pandas library helps with its easy-to-use data frame operations. Data manipulation operations such as selecting a part of tabular data, or the insertion or removal of some columns, reading from and writing to files, all become easy when a data scientist is familiar with the Pandas library. The Pandas package is a handy tool in handling large amounts of data. Pandas data frame and series data structures are compatible with popular plotting libraries, such as Matplotlib. Such compatibility made the Pandas library versatile.
The use of Pandas for initial exploration and data engineering is common. The main advantages are high flexibility in coding and convenience due to the abstraction of data manipulation concepts instead of focusing on implementing every detail for munching data. If your project requires many operations to store, manipulate, and visualize data, you will undoubtedly consider using the Pandas library. If I work on tabular data, I load the data to the main memory from the file using Pandas. Many of the times, the program I write ends with writing to a file using Pandas. Pandas is such a package that we always use but forget to appreciate its existence.
scikit-learn
scikit-learn is a machine learning library for the Python programming language. A few lines of function calls of the scikit-learn library can provide tremendously good results for many applications. Scikit-learn contains many statistical tools and machine learning algorithms for data normalization, feature selection, dimensionality reduction, classification, clustering, regression, and validation.
scikit-learn is a well-documented library. The documentation contains ample examples to clarify how to use the functions while coding. Such documentation is considered a Holy Grail for application developers. Which algorithms to use from this library to solve a specific analytic problem is something that a data scientist can decide based on her/his experience and expertise. Interpretation of the results is also critical and often requires a collaboration between a data scientist and a domain expert.
SciPy
SciPy.org provides several libraries, including NumPy, Pandas, Matplotlib, and the SciPy core package for scientific computing. I have already talked about NumPy and Pandas so far in this article and will discuss Matplotlib later. The SciPy package is loaded with mathematical and statistical functions for Fourier transformation, signal processing, image processing, linear algebra, interpolation, optimization, integration, eigenvalue decomposition, and statistical functions.
Not all data science application designers will need to use the SciPy package. This package is of tremendous help to those who use numerical analytic operations often. I frequently use SciPy for quick prototyping of solutions using mathematical optimization. SciPy is the go-to Python library for those who use Matlab for optimization and linear algebra but planning to move to the free Python platform.
Tensorflow and Keras
Tensorflow is a widely used library for designing extensive high-performing numerical solutions for machine learning. It is widely used in conjunction with Keras, facilitating designs of neural networks, including deep ones. Tensorflow can be considered as an ecosystem for machine learning modeling powered by Keras neural network functionality.
CPU and GPU computation abilities make the overall Tensorflow ecosystem a well-accepted tool for deep neural network solutions. The framework was designed for Google’s internal work first and later released to the public domain as an open-source machine learning software library. After its public release, a booming trend among data science practitioners in the industry and academia has been observed in designing deep learning solutions using TensorFlow.
Pytorch
Pytorch, Developed at Facebook, created an enormous buzz for its flexibility and modular design to work on deep neural network models. It is currently a community-driven project under BSD licenses.
While Tensorflow and Keras are still the most widely used tools for deep neural networks, the popularity of Pytorch is increasing rapidly. The use of the concepts of tensor computations, embeddings, and dynamic graphs within Pytorch makes the tool flexible for modeling different types of neural networks. Additionally, PyTorch allows GPU acceleration making it competitive with Tensorflow.
If you are already familiar with TensorFlow and Keras, you can probably take a peek at Pytorch to check if you are amazed by its features. If you are not yet familiar with Tensorflow and Keras, it is perhaps better to learn those before learning Pytorch.
Multiprocessing
The multiprocessing package in Python saves the execution time of a program by spawning processes. The tool will not automatically distribute the computation to multiple processes; rather, the programmer will design how the workload distribution will occur. Suppose there are clear tasks that can be executed in parallel with no dependency between them. In that case, we can leverage the multiprocessing package to write the program to run the tasks parallelly in multiple processes. The operating system will try to put the processes in as many CPUs as possible to speed up the computation.
Some tasks are meant to be completed serially, and some tasks can be parallelized. Depending on what tasks a data scientist is targeting, the components of the program can be parallelized to maximize CPU usage. Many times, we write our code in such a way that the program only uses one CPU. Modern computers, including our laptops, have multiple cores that can be leveraged for faster processing. Multiprocessing in Python programming aids that parallelization within the CPUs or cores of the same computer.
Mechanize
Mechanize is a Python library to create and load browser objects, submit forms, and catch exceptions thrown by the server. The library facilitates programmatic browsing. You can write a program using mechanize so that the program can programmatically log in to a website, collect data and report back to you. Mechanize is the tool that replicates human browsing in Python programming.
The use of the Mechanize API to retrieve particular information sometimes requires extensive analysis of the HTML content of the pages that the program will traverse. Initially, the programmer needs to manually inspect the HTML source of the pages to understand the underlying tags that contain the intended information pieces. Direct crawling to collect everything from all tags does not require much analysis, though.
Scrapy
Scrapy is a Python library to collect a large amount of data from websites. It is a fast framework to crawl websites and retrieve structured information efficiently. Scrapy facilitates downloading from the web and saving content on the local drives. With substantial downloading tasks, Scrapy would be the right choice for web-crawling.
Beautiful Soup
BeautifulSoup is a lightweight Python library that simplifies the web scraping process. Using BeautifulSoup, one can scrape the entire web page — titles, contents, and meta tags. BeautifulSoup provides a great way to parse HTML and XML files. It is more a parser than a downloader.
NLTK
The NLTK (Natural Language Toolkit) library in Python is a widely used platform to incorporate linguistic aspects into programs. The library has a rich set of resources and standard natural language processing (NLP) algorithms. One can use the library to process a text dataset, parse it, model it, and apply many algorithms that are already implemented in the package. From vectorization to the removal of stopwords to the creation of a frequency distribution to generating n-grams to sentiment analysis — all are provided with the NLTK library. The Valence Aware Dictionary and sEntiment Reasoner (VADER) pre-trained sentiment analysis model in NLTK is probably one of the most well-known sentiment analyzers used by researchers and social network analysts. The easiness of using the NLTK library has made it immensely popular in the NLP-community.
Regular Expression (re)
The regular expression refers to a set of rules that identify patterns of input (such as string, number, character). The regular expression library in Python helps to query patterns in the text data instead of an exact match. Some examples are — retrieving all phone numbers, zip codes, email addresses, or any format that you know might exist in the text. The re module integrated into Python provides the regular expression functionality.
The symbols used in Python regular expressions are universal across all programming languages. Learning to work with regular expressions not only helps in pattern search in the data science discipline, but it is also considered a vital skill for any computer scientist regardless of the programming language she/he uses.
Matplotlib
Matplotlib is an information visualization Python library suitable for plots in two-dimensional planes. It helps draw plots such as bar charts, scatter plots, line graphs, pie charts, contour plots, and many more from arrays. Researchers use Matplotlib generated figures in their research articles. IPython enabled editors, Jupyter Notebook, and Google Colab — all can render matplotlib drawings.
Seaborn
Seaborn is an advanced statistical data visualization library built on top of matplotlib. It provides an easy-to-use API to generate many different types of plots. The availability of many different attractive plot designs has made Searborn a popular choice for visualizing data summaries.
Plotly
Plotly is another rich visualization library in Python. It is a powerful tool equipped with interactivity. It differs from matplotlib through its interactivity aspect.
Conclusion
The developers of the Python libraries listed in this article have made it easy for anyone to quickly and effectively utilize the power of the Python programming language for conducting scientific and business research and develop analytic applications. I should mention that the mere use of these Python libraries for data science does not guarantee effective analytics. A compelling analysis of the data requires a strong theoretical background to realize all sorts of data modeling perspectives. Without robust theoretical knowledge, it won’t be easy to use the libraries fruitfully.
2 Comments
My name is Muluh Victory I am from Cameroon data science is not a course in the university in my country, but am passionate about the field, I have a good background in Computer sciences and mathematics, I don’t know how to go about my career, I need your advice because I don’t also have the means to study out of my country, thank you for your time I have learned a lot
Hello! Thank you for your message. Data Science is a growing area. Many universities might not have data science programs yet. I am glad to hear that you are passionate about data science. You can look for free online materials. I have a course — Introduction to Data Science — which I am still developing. All the videos are public and available in this playlist: https://www.youtube.com/playlist?list=PLJXHwy-4vGRZauaA3D6pCS5drNfuMMSt5
Another resource is a data science and machine learning workshop series that I am running: https://www.youtube.com/playlist?list=PLJXHwy-4vGRbLixeEJ8dQsOAeVZdBAFUz
It is immensely beneficial to use Python programming language for data science applications.
Also there are data science and machine learning courses on Coursera. Andrew Ng’s online Machine Learning course is famous and popular: https://www.coursera.org/learn/machine-learning
Thanks again for your message.