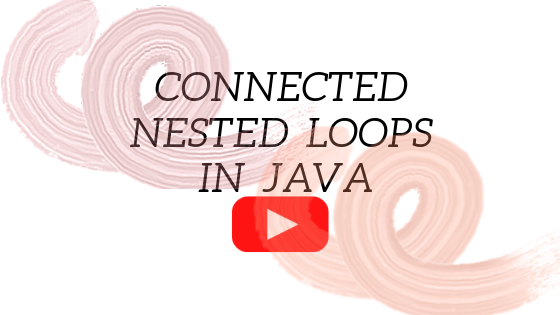
Connected nested loops in Java: Video Lecture 12
As stated in the previous video lecture, nested loops refer to the repetition of another repeated task. The nested loops we discussed in the last lecture were not dependent on each other in determining how many times a loop should iterate. In the exercise of this lecture, we demonstrate how we can ensure that the inner loop iterates a different number of times in each repetition of the outer loop.
We ended the last lecture with an exercise problem. In this lecture, we are going to solve that exercise.
Contents
Problem Description of the Exercise
Write a program using nested for loop to print the following pattern of asterisks on the terminal.
**********
*********
********
*******
******
*****
****
***
**
*
The Solution used in the Video Lecture
We wrote the following code in the video to solve the problem described above. Save the program in a file named NestedTri.java
.
class NestedTri{
public static void main(String[] args){
int i;
int j;
for (i=1;i<=10;i=i+1){
for (j=1;j<=10-(i-1);j=j+1){
System.out.print("*");
}
System.out.println();
}
}
}
The Video Lecture
The YouTube video lecture is embedded below.
Transcription of the Audio of the Video
Hi,
This is Dr. Monika Akbar. I hope that you are enjoying Java programming. In the previous video lecture, we discussed nested loops. We will discuss nested looping in this video too, but there will be some added complexity in it.
You may recall that, we provided an exercise at the end of the previous video. We will solve that exercise here today. Even if you solved the exercise, I suggest that you watch this video because, through the exercise, we will explain nested loops that are connected. The exercise today will demonstrate how the inner loop can be dependent on the outer loop. Here is the exercise problem:
Problem description
Print 10 lines, where the first line, contains 10 asterisks, the 2nd line contains 9 asterisks, the 3rd line contains 8 asterisks, the 4th line contains 7 asterisks, so and so forth. The sample output is provided below.
[Show the sample output.]
**********
*********
********
*******
******
*****
****
***
**
*
[End of sample output]
Again, by watching the video, you will learn nested looping, where the inner loop depends on the outer loop to determine how many times the inner loop should iterate.
Problem analysis
Let us analyze the problem a bit first.
Notice that the output of the problem must contain 10 lines of stars. The first line contains 10 stars. The number of stars reduces by one in the following line. The reduction continues till the tenth line.
Notice that the first line has 10 minus zero asterisks.
Line 2 has 10 minus 1 asterisks.
Line 3 has 10 minus 2 asterisks.
Line 4 has 10 minus 3 asterisks.
So and so forth.
Finally the 10th line has 10 minus 9 asterisks, which is just one star.
If the problem was to print 10 asterisks in each of the 10 lines, we could directly use the technique we learned in the previous lecture. That is, we could write two for loops, where the inner for loop prints 10 asterisks in one line, and the outer for loop repeats the inner for loop 10 times.
Output
Running this code on the terminal will give ten rows, where each row contains 10 asterisks. However, this is not what we want. We need to reduce number of asterisks in the consecutive lines.
[Show the output.]
**********
**********
**********
**********
**********
**********
**********
**********
**********
**********
[End of show the output.]
More analysis of nested loops
Notice that the outer for loop controls which line is going to be printed. That is the variable “i” controls the line number. The inner for loop is responsible for printing certain number of asterisks in a line. Now, We have to change the inner for loop because we are changing the number of stars to be printed for any line. The inner for loop is controlled by the variable “j”. We will change the inner for loop in such a way that it executes lesser and lesser number of times in subsequent lines.
Recall that, the first line should have all the 10 stars.
The second line should have 10 minus 1 stars, which is 9 stars
The third line should have 10 minus 2 stars, which is 8 stars,
The fourth line should have 10 minus 3 stars, which is 7 stars,
So and so forth.
In the code, in our inner loop, how many stars should be printed is basically controlled by this condition. Currently, the inner for loop is printing 10 stars every time because of this condition.
Now, how can we tell the program that we want to print lesser number of stars than 10, based on – in which line of asterisks – we are currently in. Notice that, the value of the variable “i” holds the information of the current line number. We can use the variable “i” to design how many less number of stars than 10 should be printed.
Controlling the inner loop using the control variable of the outer loop
Recall that, when we are in Line 1, we should print all 10 stars using the inner for loop. Therefore, we want to subtract zero from 10. Since the variable “i” has the value 1 when we are in line 1. We want to subtract i-1 from 10 when the variable “i” is 1. i-1 results in zero because variable “i” is 1.
When we are in Line 2, we want to subtract 1 from 10. In line 2, the value of the variable “i” is 2. Therefore, i-1 is equal to 1. Therefore, by subtracting i-1 from 10, we will be able to print 9 stars.
When we are in Line 3, the value of the variable “i” is 3. Therefore, i-1 will reduce 2 stars from 10, which is 8 stars.
So far, it seems putting 10- (i-1) to control the range of j will work for the first line, the second line, and the third line of asterisks. In fact, it will work for all the following lines.
Output
Let us save the file, compile it, and run the generated class file. We can see that we have the desired output, where the first line contains 10 stars, the second one contains 9 stars, the third one contains 8 stars, so and so forth.
Concluding remarks
The exercise today has shown how we can connect the inner loop with the outer one. There are many other applications that will require such connected nested loops. We will learn them over time with more complex problems.
If you have not yet subscribed to our website Computing4All.com and to this YouTube channel, please do so to confirm that you receive notifications on our new articles and videos. We will see you again in the next video. Wish you a wonderful day, evening, or night, whatever is upcoming. Thank you!
2 Comments
Please how can I join from beginning as a beginner I think have lost along the middle I just
Please visit our videos page ( https://computing4all.com/video-library/ ) . All our video lectures are listed on that page. The earliest video is at the very bottom. If you watch the videos from the bottom, you will be able to start from the very beginning of the course.