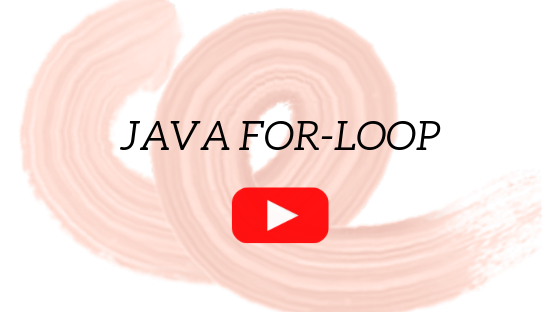
An introduction to Java for loop: Video Lecture 9
Repetition of segments of codes is an essential part of programming. Repeating the execution of a code segment, a programmer can reduce the size of the code as well as solve problems that require iterations of similar computations for an arbitrary number of times. Java provides three syntaxes for repetition: for loop, while loop, and do-while loop. This article covers Java for loop.
Contents
- 1 Java for loop
- 2 The video lecture on Java for loop
- 3 The code used in the video lecture
- 4 Exercise on Java for loop
- 5 Comment
- 6 Transcription of the Audio of the Video
- 6.1 Sample problem
- 6.2 Java for loop
- 6.3 Terminating the loop
- 6.4 Summary of the three parts
- 6.5 Let us use a Java for loop in our code
- 6.6 Values of “i” in each iteration
- 6.7 Tracing the program
- 6.8 i=0
- 6.9 i=1
- 6.10 i=2
- 6.11 i=3
- 6.12 i=4
- 6.13 Our example loop terminates when i==5
- 6.14 Concluding remarks regarding Java for loops
Java for loop
Java for loop is is a powerful syntax to repeat segments of code. It provides integral mechanisms to use variables to control a looping. The “for” syntax itself has three parts: initialization, condition, and change (modification of variables.) These parts are seperated by two semicolons. The basic for-structure is provided below.
for (initialization; condition; change){
// Write the code you need to repeat.
// This is the scope of the for loop.
}
The first part, initialization, is executed only once. It executes before anything else regarding the for-loop has been executed.
The second part, condition, executes each time the execution attempts to go insde the scope of a for-loop. The execution goes inside the loop if the condition results in true, otherwise it terminates the loop.
The third part, change, executes at the end of each iteration. Generally, increment or decrement operations on variables are applied in this part.
All the three parts are optional. Java for loop is a powerful syntax because a programmer can use it quite flexibly and creatively. In fact, knowing just “for loop” from the looping control statments is good enough. A programmer can skip while loop and do-while loop pretty easily, if she/he learns the for-loop well. (If you are reading this page for a coursework, I must tell you NOT TO SKIP while loop and do-while loop.)
The video lecture on Java for loop
The following video provides great details on how to use Java for loops. It is a great introductory material. The last part of the video contains a tracing of the program to visually explain how a Java for loop works.
The code used in the video lecture
Here is the code we used in the video lecture. Save the code in a file named MyCode.java
.
class MyCode{
public static void main(String[] args){
int i;
for (i=0; i<5; i=i+1){
System.out.println("Hello Java!!!");
System.out.println(i);
}
}
}
Exercise on Java for loop
Use Java for loop in solving all the problems below.
- Write a program to print your name twenty times.
- Write a program that sums up all the integers between 1 and 100 (including 1 and 100.) Report the final summation on the terminal.
- Write a program that prints all the odd numbers between 0 and 50 on the terminal.
Comment
Please feel free to send us your code in solving the exercises above. Write your questions in the “Comments” section below. We will be happy to help you in problem-solving.
Transcription of the Audio of the Video
Hi, this is Dr. Shahriar Hossain. Have you ever thought about how can we repeat segments of our code for a certain number of times? Like most standard programming languages, Java has three different syntaxes for repeating a segment of code. These syntaxes are called the “for loop”, the “while loop”, and the “do-while loop.”
Today, we are going to learn the “for loop.”
Sample problem
To explain a sample problem, let us write a program. The problem is to print “Hello Java!!!” five times. Based on topics we have discussed so far in this lecture series, we would write five System.out.println statements to print “Hello Java!!!” five times. If we have to write “Hello Java!!!” one hundred times, we have to write one hundred System.out.println statements. We might even have situations, where we have to repeat the same statement a thousand times. It is not quite practical to repeat statements so many times in the same program. To solve this issue, all languages provide looping.
Java for loop
Java’s “for” control statement has three parts. The first part is initialization. This part is executed once before entering the scope of the for loop. The second part is condition check. As long as this condition check is true, the execution will go inside the scope of the for loop. The third part is something that is automatically executed after each iteration of the scope.
All these three parts are separated by two semicolons, within the parentheses. Now notice that if you initialize a variable in the first part, and increase the variable in the last part, then you can put a condition in the second part to allow the execution to go inside certain number of times. For example, consider that you have a variable named “i”. You initialize the variable “i” to 0. Then you, say in the third part, i=i+1. The third part executes automatically after the execution of the segment scoped by the for loop. Therefore, at the end of each iteration i will automatically be incremented by 1.
You are starting the loop with i=0. After executing the code inside the scope of the for loop, the value of i will become 1 because i=i+1 will execute. In the next iteration, I will remain 1; then at the end of the iteration, i will become 2. This will continue for ever, unless you put a condition in the second part of the three parts of the for loop.
Terminating the loop
You can tell when to terminate the loop. In this example, you can tell “when to stop” based on the value of the variable i. Again, the second part should hold a condition; that is, it should result in a Boolean value. Before executing the scope, the program will check the second part if it is true. If it is not true, then the program will not go inside the loop.
If you type i<5, then it means as long as the value of “i” remains smaller than 5, the execution should go inside the scope of the for loop.
Summary of the three parts
Again, the first part is the initialization and executed only once. The third part is executed after every iteration. The second part is checked for “true” every time the execution attempts to go inside the scope of the for loop.
Since, we have i=0 in the first part, i<5 in the second part, and i=i+1 in the third part, this particular for loop will go inside the scope five times. We will discuss more on this soon in this video.
Let us use a Java for loop in our code
For now, let us change our code with five System.out.println statements so that our code contains only one System.out.println statement, but we repeat that statement five times using a for loop.
I am declaring an integer variable named “i”. I will use this integer variable to control the for loop.
Based on the discussion so far, all we have to do is, write a for loop, in which the first part will contain the initialization. I am putting i=0. In the second part I am putting i<5. In the third part I am writing i=i+1; Notice that, starting from 0, there are five integer numbers that are smaller than 5. Therefore, the loop will execute five times.
Let us save the file, compile, and run it. The program prints “Hello Java!!!” five times.
Values of “i” in each iteration
To check the value of the variable “i” during each of the five iterations, we can write another System.out.println statement, just to print the value of “i”. Save the file, compile, and run it.
Notice that after printing each of the “Hello Java!!!” phrases, we have a number printed in the next line which corresponds to the value of “i” in that particular iteration.
Let us trace the execution of the program.
Tracing the program
I am using a software to trace the program. You are already familiar with manual tracing from the previous video. In this video, I am doing tracing using a software named Netbeans to trace the execution of the code I have written. You do not have to use Netbeans at this point; I am using Netbeans because it gives a good visual illustration of what I am teaching today.
The light green highlight shows where the execution is right now.
I am going to track two items during this tracing. One is the value of the variable “i” and the other is the Boolean value of “i<5”. I have the output-terminal too.
i=0
Now, the program is in a state where it has created memory space for the variable “i”. The execution is currently in the line where we have the syntax “for”. It has not yet executed the initialization i=0. The first thing the computer will do is execute, the initialization i=0. Then it will check if i<5. Because the variable “i” is zero after the initialization, 0<5 will be true. The execution will go inside the scope of the for loop. Notice that we have two System.out.println statements inside the scope of the for loop. The execution point is now on the first System.out.prinln statement.
At this point, the variable “i” is zero, as you can see here. Also notice that i<5 is true. This is the reason the “for” control statement has allowed the execution to go inside the scope of the for loop. If we execute the current line, which is System.out.println (“Hello Java !!!”), then “Hello Java !!!” will be printed on the terminal. Our execution point has moved to the next line. Notice that “Hello Java !!!” has been printed on the terminal.
Again, the current value of the variable “i” is 0. Therefore, if we execute the current highlighted line, 0 will be printed on the terminal and here it is. 0 is printed on the terminal.
i=1
After 0 is printed on the terminal, the “for” control statement has executed the scope for the current iteration just once. Now, the third part of the for statement will execute. The third part is i=i+1. Again, the third part is executed at the end of each iteration. Once the variable “i” is incremented by 1, “i” becomes 1 because 0+1 is 1. At this point we are in the second iteration of the scope of the for loop. Notice that i<5 is a true statement because the variable “I” is 1, and 1 is smaller than 5 is a true statement. This is the reason why the execution was able to enter the scope with 1 as the value of i. We print “Hello Java!!!” for the second time on the terminal. Then we print the value of the variable “i”, which is 1 at this point.
i=2
Once the content of the variable “i” is printed on the terminal, i=i+1 will be executed and I will become 2. Since 2<5 is a true statement, the execution will go inside the scope. The first System.out.println will be executed. The execution will now go to the second System.out.println statement. Since the value of “i” is 2, the program will print 2. After printing 2, the third part of the for loop will be executed.
i=3
Therefore, the value of the variable “i” will become 3. Since 3<5 is a true statement, the execution will go inside the scope of the for loop. “Hello Java!!!” will be printed for the fourth time. The value of the variable “i”, which is 3 will be printed.
i=4
The value of the variable “i” will now become 4. Now, 4<5. Therefore, the execution will go inside the scope of the for loop. “Hello Java !!!” will be printed for the fifth time. In the next System.out.println statement 4 will be printed because the variable “i” now contains 4.
Our example loop terminates when i==5
At this point, the third part of the for syntax, which is i=i+1, will be executed. The variable “i” will become 5. Now 5<5 is a false statement. Therefore, the execution will not go inside the for loop anymore. Once the second part of the for loop becomes false, looping is terminated. The execution will jump what we have after the for loop. In our case, it is the closing curly bracket of the main method. Notice that, at this point the variable “i” is 5, and therefore the condition i<5 is false. This is the reason the for loop terminated.
By this time, we have printed “Hello Java!!!” five times on the terminal.
Now, won’t you be able to print “Hello Java!!!” ten times, on the terminal. Or say, print your name on the terminal fifty times, using a for loop? I’m sure you will.
Concluding remarks regarding Java for loops
Now that we can repeat code segments, we can write complicated codes. We can sum up all integers between 0 to 100. Or, we can find if a number is a prime number or not. Note that, you can write arbitrary number of lines inside the scope of the for loop. Those lines may contain anything including if-else if statements, or oven other for loops. More on this in the upcoming videos. If you have not already subscribed to our website Computing4All.com or to this YouTube channel, please do so to receive notifications on our new posts.
Thank you for watching the video. Hope to see you soon in in our video lecture series.