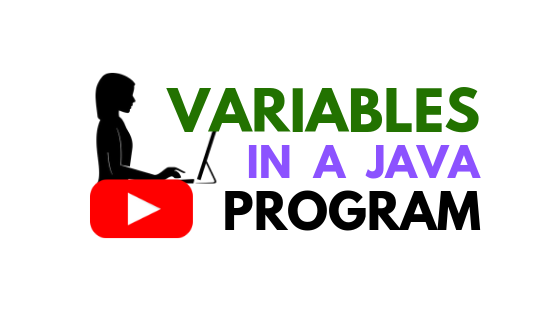
An Introduction to Variables in Java: Video Lecture 2
I am writing this article today in support of the video I made on Variables in Java. The video describes a little bit of theory and then demonstrates how you can use multiple variables in your program. I used simple applications — addition and subtraction of two numbers — to describe how to use variables in Java. The target was to make the video suitable for learners who have just started to gather study materials to learn the Java programming language.
Watch the video and practice at the same time. Pause and repeat as required. Learning will remain incomplete without practicing the content of the video. Please note that learning a programming language requires practicing. It is like learning to swim. Reading a book on how to swim helps in understanding the principles, but no one can become a swimmer without really attempting to swim.
Contents
- 1 The Video: Variables in Java
- 2 Additional Resources
- 3 Exercise
- 4 COMMENT
- 5 TRANSCRIPTION (OF THE AUDIO OF THE VIDEO)
- 5.1 What you will learn today
- 5.2 Thank you for your feedback
- 5.3 What is a variable?
- 5.4 There can be many types of data and hence variables
- 5.5 The concept of a container
- 5.6 What types of variables do we discuss in this video?
- 5.7 Writing MyProg.java
- 5.8 Declaring variables
- 5.9 The assignment operator
- 5.10 Displaying the summation: The wrong way
- 5.11 Displaying the summation: The correct way
- 5.12 Congratulations
- 5.13 Copying the result to a variable
- 5.14 Subtraction
- 5.15 Printing numbers without context is boring
- 5.16 How to include context text in the terminal output
- 5.17 An introduction to “double” numbers
- 5.18 Conclusion of the video
The Video: Variables in Java
Variables are in the core of any programming language. Learning to handle variables in Java will aid in learning any programming language later.
Additional Resources
The following two pages provide further details on variables in Java, especially regarding the naming convention of variables and basic data types.
- Variable naming convention: Please read the “Naming” section of the link.
- Primitive data types: The link will give you an idea on what general variables are there in Java, other than
int
anddouble
that we covered in the video.
Exercise
If you want to practice more on the use of variables in Java, here is an exercise for you.
Write a Java program that has at least two double
variables named num1
and num2
. Assign any decimal values to num1
and num2
. Then on the terminal, display the addition (num1+num2
), subtraction (num1-num2
), multiplication (num1*num2
), and division (num1/num2
) results. When showing a result, put a text first and then put the result. For example, for the summation, if the summation is 35.8, then display the following sentence: The Summation is 35.8. That is, do not just print the results; put some context with each result.
COMMENT
We will come back with the next video in this video lecture series in a few days. We will cover more on variables in Java. To stay in touch, please subscribe to Computing4All.com. Enjoy!
TRANSCRIPTION (OF THE AUDIO OF THE VIDEO)
Hi,
I am Dr. Shahriar Hossain. I am here today to explain and help you practice the concept of variables in Java programming language. In our previous video, I explained how to write, compile, and run your first Java program. We are advancing toward more and more rigorous programming concepts. Although I am teaching programming language Java in this video lecture series, the concepts will help you learn other programming languages. Overall concepts are similar between core programming languages.
My target is to cover the theories as well as to help you practice some applications. In each video, I will try to cover the theory and then share the view of my desktop with you so that you can see how I write a program to practice the theory.
What you will learn today
Today, you will learn the concept of variables. The concept of variables is in the core of any programming language. You handle data through the use of variables in your programs. Today, we will see how you can add two numbers stored in two variables. I teach very slow so that everyone can cope with any new concept I cover. There is no point of making these videos if I cannot explain the concepts well to you in a meaningful way.
Thank you for your feedback
After publishing the previous video, I received a message from one of the viewers stating that she/he was not able to hear what I was saying because the sound-level was not adequate. I really appreciate any feedback. I checked my microphone. I figured that the problem was not with the microphone. The problem was and still is me. I do not and probably cannot speak loudly. As a solution to the problem of low-level audio, I will amplify my voice using a synthesizer before publishing the video. Thanks to the person who provided the feedback.
Please keep sending us feedback regarding any of the videos we make. You can provide feedback through Computing4All.com or directly from YouTube comments section below the video.
Let us talk business now. Let us talk about what variables are in a Java program?
What is a variable?
A variable in a Java program or in any program written using any standard programing language refers to a content kept in memory space. When I said “Content” I meant “data”. For example, a number is a data content. A person’s name can be considered a data content. You want your program to remember some data content throughout the runtime of the program. Anything that you want your program to remember has to be stored in variables.
Now, where are these variables kept? In a computer, when you run your program, the variables are kept in the main memory of the computer. The main memory of the computer is commonly called RAM, which is short for Random Access Memory. Therefore, whenever you run a program the program uses some space of the RAM to store things it has to remember. When you write the program, you provide instructions on how to use the variables.
There can be many types of data and hence variables
Now, think about how many types of data you may have. Actually, there can be many types of data. For example, some data can be numeric, like age, salary, price, or any countable amounts. Some data types may not be numeric. For example, names of people, address, book title, these are non-numeric data. Such non-numeric information pieces, which require natural language are generally called strings.
So far, we have discussed numeric data and strings. There is another type of data, which is just one letter, for example, sometimes on calendars, you see the days of the week stated as S for Saturday, S for Sunday, M for Monday, that is the first letter of the day of the week is used to represent the day. Such one letter symbols are called characters. Therefore, ‘a’ is a character. ‘b’ is another character, so and so forth.
We have talked about three types of data. (1) Numeric, (2) Non-numeric String, which has more than one letter, and (3) characters containing just one letter.
The concept of a container
In this particular video, we will only practice two of the numeric data types. Now let us think about numeric data types. Some number are small; some are big. For example, the age of a person. How large this number can be in reality? Probably, age can be 100 years or at most 120 years. Now, consider the yearly income of people in the United States. It can be as small as zero. It can be as large as a few million. Therefore, a variable that stores age of people can have a way lower capacity than a variable that stores the income of people.
You can consider a variable as a container. If you need to carry one liter of water from here to there, you do not use a barrel that can hold fifty gallons of water. You will probably prefer to use a bottle instead of a barrel. On the other hand, if you need to move 40 gallons of water, you will probably use a large barrel instead of using a small bottle because to move 40 gallons using a small bottle you will have to back and forth several hundred times.
Memory space in a computer is limited. Therefore, we use variables wisely. I mean, we do not have to be wise at this point because our programs are quite small, but at some point, we will have to make sure that we are using the correct type of variable for the right kind of data.
What types of variables do we discuss in this video?
Today, I am going to cover two types of numeric variables. The first one is called Integers, and the second one is called real numbers or double numbers.
An integer variable can hold numbers that do not have any decimal point. For example, 5, or, 10, or, the number 20. You cannot keep the number 3.6 in an integer variable. To hold numbers that have decimal points, you need a double or float variable. I will demonstrate how we can use integer and double variables in Java.
Now, let us go to the computer and take a look.
Writing MyProg.java
At first, I will write the structure of the program. As I said in the previous video, I use a simple text editor to write my programs. I am using XCode now on a Mac. You can use notepad on a Windows machine. The name of the file I will work on is MyProg.java. As you know from the previous video, the name of the class has to be MyProg if the finename is MyProg.java.
Inside the scope of my MyProg class, I am writing the main method. The main method has the combination of syntax “public static void main” and String start and end square brackets within the parentheses. Then write args.
Then mention the scope of the method by providing a set of start and end curly braces.
Everything we will write today will be inside the scope of the main method.
Suppose, the problem we are going to solve today is the following. How can we add two numbers and display the result?
Declaring variables
The first thing to do is — declare two variables for the two numbers. That is we will store each number is a variable.
We are going to use an integer variable for each of these two numbers.
This is how we declare integer variables.
The first thing to say is the data type then we have to give a name to the variable. The data type Integer is expressed by just “int” in Java.
I type int then I provide a variable name. The name of my variable is “a”.
We could name my variable “aa”, or anything we want, within certain restrictions. One convention is a variable name should start with a letter. A restriction is, you cannot use a reserved word as your variable name. For example, you cannot use the word “class”, or “public” as your variable name because these words are already reserved by Java to be used in the code.
Anyway, I type int then I put the variable name “a” then I put a semicolon. When I put a semicolon, the Java virtual machine detects it as an end of one instruction. Therefore, int a semicolon is a complete instruction. When running the program and running this specific line, the Java Virtual Machine will create a variable, which has the data type of Integer and the name a.
Now, let us create a second variable. We will keep the second variable of the same type as a. We will give the second variable the name “b”.
Therefore, we write int b and then put a semicolon. Now we have two variables. That is, Java has created two variables where we can put numbers.
The assignment operator
Let us put numbers in each of these containers a and b. This is how we are going to do it.
We just write a equals 10 and put a semicolon.
The equal symbol is called an assignment. The right side of the assignment is executed first and then the value is dropped inside the variable in the left side. I should mention to clarify that the left side of an assignment operator can have only one variable.
Instead of writing, a equals 10, we could write a equals 8 plus 2. In that case, the virtual machine will execute the right side of the assignment operator first, which would result in 10 and then copy the value 10 in variable a. That means, the container a will contain 10 after the execution of this line. By container a, I meant variable a.
Now, let us put another numerical value in the variable b. We write b equals 20 to put 20 inside variable b.
When we will run the program Java will come to the first non-empty line inside the main method. It will create the integer variable a. Then, it will create the integer variable b. Then it will put 10 inside variable a. It will put 20 inside variable b. Notice that we have not displayed anything yet. Suppose, we want to display the summation of variable a and variable b on the screen.
Displaying the summation: The wrong way
We might remember from the last video lecture that we use System.out.prinln to print whatever we want to print on the terminal. So I am writing the System dot out dot println instruction here. A note from the last video – we write whatever we want to write inside quotes. So, I am writing inside quote “a plus b”. Soon, we will see that it is not the right way to print the summation of a and b. Let us compile and run the code. You will see what I am saying.
I use Javac to generate the class file. The command is javac MyProg.java. MyProg dot class is generated. Now, I will run the class. The command is java MyProg. After I run it, I should see the summation of a and b on the screen. Oops. Wrong. I see the text “a+b” is written. I wanted to see the summation of a and b. Therefore, I have written something wrong in my code. Let us go back to the code.
Displaying the summation: The correct way
Notice that I write a plus b inside double quotations. Whatever is written inside quotations, will be printed on the terminal and that is what happened in the output of the program. The text “a+b” was printed. This is what we do to really print the summation of the variables. We just remove the quotations. When we remove the quotations, Java will execute whatever you have within the parentheses. You have a plus b within the parentheses. a is 10 and b is 20. The summation of 10 and 20 is 30. Therefore, System.out.println will print 30 on the screen. Let us compile the java file again. Let us run the code now. You can see that the result 30 is printed on the terminal now.
Congratulations
If you are practicing with me right now and if you have come this far of this video, many many congratulations on the successful use of two variables using a Java code. You will be surprised to find what you can do with this knowledge. You can practically write a calculator program with the knowledge you have gained so far. Let us go with a small example today. Let us go to the code.
Copying the result to a variable
We will modify the code a little bit. Let us introduce another variable named result. This variable result will contain the summation of the two variables. I am writing int result and then I am putting a semicolon. Therefore, when this line will be executed, there will be a variable named result, which may contain any number. Now, I am writing result equal a plus b. Then put a semicolon.
When this line is executed, the right side is executed first and whatever is the outcome of the right side is copied to the variable in the left side.
In the right side, the virtual machine will find a, which is a variable then plus, then another variable b. Java will take the content of “a”, which is 10. Then it will take the content of “b”, which is 20. Then Java will sum up the contents, which will be 30. Now, Java will take 30 and put it in the variable at the left side. Therefore, the variable named “result” will contain 30 after this line is executed.
Now, in the System.out.println part instead of writing a plus b, I will just write result. Therefore, System.out.println will print whatever content I have in the variable named “result”. Hence, 30 will be printed.
If we compile the code and run it again, we will see that the result is 30, as expected.
Subtraction
Now, we will try to do a subtraction, but I will use the same variable “result” to store the subtracted value.
One thing to notice here is that after we print the summation using System.out.println method, we can reuse the result variable to hold the subtracted value. The computer executes the program line by line in a sequence. After executing the summation, we want to print a subtraction result. Therefore, after the summation result is printed we, write result equals a minus b. Then put a semicolon. Remember that a is 10; b is 20. Therefore, the subtraction result will be negative 10. Now, we will print the result using another System.out.println instruction.
Let us compile our MyProg.java file. Then run the program. Now notice that the first line contains 30 and the second line contains negative 10. The output displays addition and subtraction of two variables a and b, where a is 10 and b is 20.
Printing numbers without context is boring
Now, notice one thing that the numbers are directly printed, without any context. I want to at least say, “The summation is 30” instead of just printing 30. And, “The subtraction result is -10” instead of just printing negative 10 on the terminal.
To accomplish this, we have to work on our System.out.prinln instructions. We already know that, when we want to print plain text, we write the plain text inside double quotes and put it in the parentheses of System.out.println statement. We will do that right now.
How to include context text in the terminal output
With the System.out.println method, for the summation result, I am writing “The summation is ” then I want Java to put my result. I cannot write the variable name “result” inside the quotations because in that case output will be “The summation is result”. What we will do is we will keep the variable name “result” out of the quotations. We will tell java to include the content, 30, of the variable after this text.
To concatenate two texts or one text and one number, we just use the plus symbol. In this case, we tell the computer that we want to include the number in the variable “result” after the text “The summation is ”. Notice that I have written, in quotation “The summation is ” then I typed a plus symbol and then I write the variable result. When printing, the System.out.prinln method will replace any variable name with the content of the variable. As a result, the printed item will be “The Summation is 30.”
I am making a similar modification for the subtraction by adding “The subtraction result is ” before the variable result. I have used the plus symbol between the text and the variable name, like the previous line.
Now, compile the file and run it. We have the desired output. The first line contains “The summation is 30” and the second line contains “The subtraction result is -10.”
An introduction to “double” numbers
Now that you have learned about variables, you can do a few tests. For example, can your code handle decimal numbers?
Let us check. Let us change the value of the variable a to 22.5. Now let us see what happens if we compile the code. We receive an error. The error states that the variable a and the number 22.5 have something to do with “incompatible types.” The reason of the error is, data type “int” can only handle integers, that are numbers that do not have any decimal points. A type that can handle real numbers or, numbers with decimal points is called double. Let us change the integer type to double. Now, let us change the type of b as well to double. Therefore, a and b are both now capable of holding real number, that is, numbers with values after the decimal point.
We need another modification. The variable “result” should be “double” just like a and b because the summation or subtraction it will hold now is also a real number with a decimal point.
Let us compile and run the program now. Notice that the first line now contains “The summation is 42.5” because a was 22.5 and b was 20. The second line contains “The subtraction result is 2.5”, which is correct for “a” equal to 22.5 and “b” equal to 20.
You can now change the values of a and b, then compile and run the program to get a feeling about how your program with multiple variables work. The website computing4all contains exercise associated with today’s video lecture. The more you will play with your code the more you will become skilled.
Conclusion of the video
As I said in the previous video, practice makes women and men great in programming. Therefore, keep practicing. Too keep in touch, subscribe to our website computing4all.com and also subscribe to our YouTube channel. I will see you in the next video. Happy programming!
4 Comments
Your lectures are well understood. Many thanks.
I am glad that you liked the lectures. Thank you for watching and your comment.
public class Exercises3{
public static void main(String [] args){
double num1 = 10.2;
double num2 = 20.5;
double result1 = num1+num2;
double result2 = num1-num2;
double result3 = num1*num2;
double result4 = num1/num2;
System.out.println(“The Summation is : ” +result1);
System.out.println(“The Substraction is : ” +result2);
System.out.println(“The Multiplication is : ” +result3);
System.out.println(“The Division is : ” +result4);
}
}
Finish Exercise.Thanks for teaching.
Awesome! Thank you for posting your exercise solution. The code looks great. Please let us know if you have any questions. I wish you a wonderful weekend.