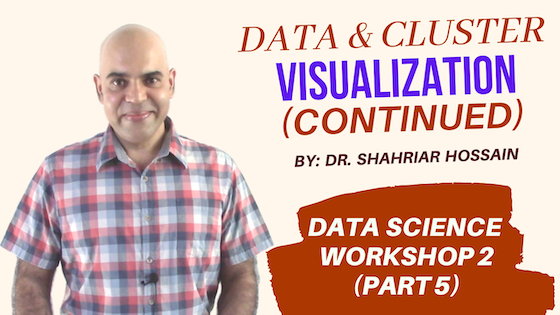
Data Science Workshop 2 (Part 5): Data and Cluster Visualization (Continued)
As I was saying in the previous part, we will not get an interactive graphical interface if we use Google colab. That is why I am using regular Python on my computer.
When I use Python, my program needs to display the figure on a canvas. Unlike directly trying to display fig on the notebook like what we do on Google colab, we will use a method to display the plot on a graphical interface. The entire data and cluster visualization technique is demonstrated in the following YouTube video.
The Python code that I wrote in the video is as follows:
import matplotlib.pyplot as plt from sklearn.cluster import KMeans import numpy as np import matplotlib.cm as cm data = np.array( [[20, 25, 18], [3, 2, 3], [6, 4, 5], [24, 21, 19], [22, 24, 21], [18, 20, 21], [4, 2, 3], [1, 5, 2], [19, 20, 19], [23, 19, 22]] ) km = KMeans(n_clusters=2).fit(data) print(km.labels_) fig=plt.figure() ax = fig.add_subplot(111, projection='3d') ax.scatter(data[:,0], data[:, 1], data[:, 2], c=km.labels_, cmap=cm.rainbow) ax.set_xlabel("Feature 1", fontsize=16) ax.set_ylabel("Feature 2", fontsize=16) ax.set_zlabel("Feature 3", fontsize=16) plt.show()
Please write the code in a file with .py extension and run it from the command prompt to view the interactive display. The video explains how to run the program.