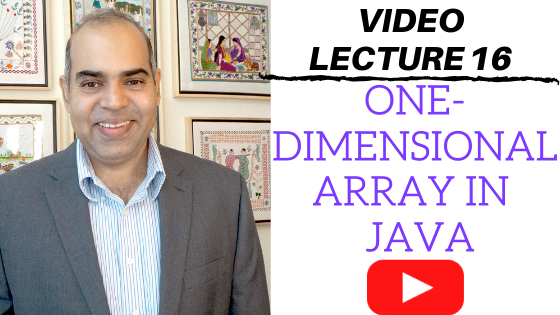
Single dimensional array in Java: Video Lecture 16
A single dimensional array or a one-dimensional array can hold more than one element. Using an array, one can avoid the use of too many variables to store the same data types. As an example, a programmer can use an array variable to refer to the GPA scores of many students. In this article, I will discuss how to declare, initialize, and use a single dimensional array.
The items we are going to discuss in this article are as follows:
- Why do we need an array variable?
- How can we declare an array of a primitive data type?
- How can we initialize the array with values?
- Example usage of an array: Sum the numbers stored in an array.
A video to support the discussions of this article is provided below.
Contents
Why do we need an array variable?
Suppose that we need to store how many miles each of ten people walked in a month. We will use an integer number for the mileage of each person. In our code, we can write ten variables for ten persons. Such as,
int milep1;
int milep2;
int milep3;
int milep4;
int milep5;
int milep6;
int milep7;
int milep8;
int milep9;
int milep10;
Let us assume that we already know what mileage values should be in the variables. Ten people walked 20, 22, 40, 18, 23, 25, 29, 35, 21, and 17 miles, respectively. Therefore, we can assign the values during the declaration time of the variables.
int milep1=20;
int milep2=22;
int milep3=40;
int milep4=18;
int milep5=23;
int milep6=25;
int milep7=29;
int milep8=35;
int milep9=21;
int milep10=17;
The problem of using too many variables
One problem of using too many variables is that our code cannot be easily generalized. That is, the program will always work only for ten people because it has ten fixed variables to store the monthly mileage of ten people.
What would happen if we have 20 people? We will need to write another program that has 20 variables. What if we have 1000 people? We will need to write that has one thousand variables to store the walking distance of one thousand people.
Writing variables for so many people is not feasible.
A single dimensional array in Java
We can consider a single dimensional array to be a row of consecutive variables — each cell in the row stores one piece of information, such as the monthly walking mileage of a person. Therefore, instead of creating ten different variables for the walking mileage of ten different people, we will create one array that contains ten cells.

Let us say, the name of the array is “mileage”. The content of the array would be the numbers 20, 22, 40, 18, 23, 25, 29, 35, 21, and 17, that we wan to store for ten people.
We refer each of these cells by a location number. It is intuitive to call these cells the first cell, the second cell, the third cells, so and so forth up to the tenth cell.
In Java, the first cell has a location number of 0.
The second cell has a location number of 1.
The third cell has a location number of 2.
So and so forth.
Note that the tenth cell of this array has a location number of 9.

Each location number or a position in an array is called an index. That is, indices start from 0. The last index of the “array” is equal to the “length of the array” minus 1. Therefore, in this array, the last index is 10-1=9.
How can we declare an array of a primitive data type?
One can declare an array of any data type using the following syntax format.
dataType[] variableName;
In place of the word dataType
, we can write whatever primitive data type is appropriate for our program. Some examples of primitive data types are int
, double
, long
, byte
, float
, and char
. In place of variableName
, we can write whatever valid name we want to give our variable.
For our running example of walking-mileage, we will use an integer array. Therefore, the declaration will be:
int[] mileage;
Example declarations of some other primitive data types are as follows.
For an array, named mydoublearray
, of double-size elements, we use:
double[] mydoublearray;
For an array, named mylongarray
, containing long integers, we write:
long[] mylongarray;
That is, we can directly use the element data type and put the start and end square brackets and then provide the array name to declare an array variable.
The difference between a primitive variable and an array variable
An array variable is slightly more complicated than a regular variable. A regular primitive data type variable contains one value only. In contrast, the array variable does not hold one element. Instead, an array variable refers to the starting of several consecutive elements.
If we consider an array a house, the cells are analogous to the rooms of the house. The index refers to a room. The array variable-name refers to the address of the house.
Similar to the house scenario, the array variable mileage
we declared for our running example is an address in the main memory referring to the beginning of our array. That means we still have to create the cells starting from that address.
Allocating space for the content of the one-dimensional array
To create the actual space to hold a certain number of integers, we have to specifically mention how many cells of integers we want in the array.
The command involves a syntax called new
. The following line demonstrates how we can allocate memory for ten cells of integers using the new
syntax.
mileage= new int[10];
The syntax new
reserves a certain number of cells for a variable. “mileage=new int[10];
” will reserve space for 10 integer cells for the array variable mileage
.
Instead of writing “int[] mileage;
” in one line and then the “mileage=new int[10];
” syntax in a second line, we can directly declare and apply the “new” syntax in the same line like the following one.
int[] mileage= new int[10];
Initially, each cell of a newly created array contains a zero as its content. Afterward, we can change the content.
How to initialize an array
How can we access each cell of the array? Say, we want to put 20, 22, 40, 18, 23, 25, 29, 35, 21, and 17 respectively in the cells of the array mileage
.
A cell of an array can be accessed by using its index number.
If we want to copy 20 in the first index of the mileage
variable, we will write the following code.
mileage[0] = 20;
This command will copy 20 from the right side of the assignment operation, and put it in the index location of 0 of the mileage
variable. Similarly, we can put rest of the numbers in the desired locations using more assignments.
mileage[1] = 22;
mileage[2] = 40;
mileage[3] = 18;
mileage[4] = 23;
mileage[5] = 25;
mileage[6] = 29;
mileage[7] = 35;
mileage[8] = 21;
mileage[9] = 17;
The commands have copied content in our entire array. That is, we can access the cells of an array, by writing the index in square brackets after the array name.
How can we print the elements of an array using a loop?
To print all the numbers in the array, we will go over all the indices. Remember that indices start from 0 and end at “length of the array” minus 1. Therefore, to go over every index, we will need to write a loop that iterates over 0 to the length of the array minus 1.
We can retrieve the length of the array by writing myArray.length
, where myArray
is the name of the array variable. For our running example, mileage.length
retrieves the length of the array.
The following code will print all the numbers in the array on the terminal.
class ArrayExample{
public static void main(String[] args){
int[] mileage;
mileage[0] = 20;
mileage[1] = 22;
mileage[2] = 40;
mileage[3] = 18;
mileage[4] = 23;
mileage[5] = 25;
mileage[6] = 29;
mileage[7] = 35;
mileage[8] = 21;
mileage[9] = 17;
int i;
for (i=0;i<=mileage.length-1; i=i+1){
System.out.print(mileage[i]+" ");
}
System.out.println();
}
}
In the code above, we have a for-loop. We use an integer variable named i
that moves from one index to another. i
starts at 0. i
is always smaller than or equal to 9 for our array mileage
. We write i<=mileage.length-1
in the for-loop. mileage.length
is 10 in our running example. Therefore, mileage.length-1
is 9. That is, inside the for-loop, i
will vary from 1 to 9.
Of course, we will go to all consecutive indices, so we write i=i+1
, in the third part of the for-syntax, indicating that we need the virtual machine to increment the value of i by one at the end of each iteration for the loop.
Inside the loop, all we have to do is to write a System.out.print
statement. Within the parentheses, we have to print the content in the ith index of the array mileage.
That is, we print the content of the cell in index i
of the array mileage[i]
. We also include a space character after printing mileage[i]
, so that consecutive numbers are separated when printed.
Outside the loop, we write a “System.out.println();
” statement. This statement will ensure that there is a newline after the numbers are printed. That is, the command prompt will appear in another line, not in the same line where the numbers are printed.
After compiling and running the program, we will have all the numbers printed on the terminal.

Direct initialization of an array
We can initialize the array directly during the declaration time. There is a way to initialize during declaration, which implicitly has a new
syntax. Instead of writing the initialization lines with ten assignments, we could declare the array variable and initialize it in one line, using the following syntax.
int[] mileage= {20, 22, 40, 18, 23, 25, 29, 35, 21,17};
That is, we can put all the numbers separated by commas within a pair of start and end curly brackets to avoid writing ten additional lines of code. The modified code is the following one.
class ArrayExample{
public static void main(String[] args){
int[] mileage = {20, 22, 40, 18, 23, 25, 29, 35, 21, 17};
int i;
for (i=0;i<=mileage.length-1; i=i+1){
System.out.print(mileage[i]+" ");
}
System.out.println();
}
}
The output of the program will remain the same.
Although such direct initialization of arrays is popular in academic settings, in practical programming, there is not much scope to leverage the benefit of direct initialization. In a real-life program, you will ask the user for how many numbers there should be in the array. Then you will use the new syntax to create an array of that size. Then you will ask the user to enter the content of the array.
Since we are still learning how to use an array, we will keep using such direct initialization for convenience.
Example usage of an array: Sum all elements
As an example, we will sum up all the elements of the array. Notice that we can use a similar loop to the one that we used to print the elements.
We will create a variable named sum
, which will have zero in it in the beginning. We will go over every element using the index, and add the corresponding content in the array to the sum
variable.
The updated program below includes the code for summing up the elements in the previous code.
class ArrayExample{
public static void main(String[] args){
int[] mileage = {20, 22, 40, 18, 23, 25, 29, 35, 21, 17};
int i;
for (i=0;i<=mileage.length-1; i=i+1){
System.out.print(mileage[i]+" ");
}
System.out.println();
int sum=0;
for (i=0;i<=mileage.length-1; i=i+1){
sum=sum+mileage[i];
}
System.out.println("Total mileage: "+sum);
}
}
The second for loop in the code is for the summation of all the elements. Before the second loop, we declared the integer variable sum. Once we are inside the loop, we deal with the ith index. Our target is to add the content of the i
th index of the mileage
array to the sum
variable.
As an example, when i
=5, inside the loop sum
should already hold the summation result of all the contents between indices zero to four.
To add the content of the i
th index, with whatever content we have in the sum
variable, we write sum+mileage[i]
. This is written on the right side of the assignment. The summation result is put back to the variable sum
by writing “sum=sum+mileage[i];
” inside the loop.
After the loop ends, we print the value of sum
, which contains the summation of all the elements of the array variable mileage
.
The program will print total mileage walked by ten people. Here is the screenshot after running the updated program.

The program prints the numbers in the array. Then it prints the summation result.
Exercise
- Write a program that starts with an array of ten integers. Print all the integers on the terminal. Then print the biggest number in the array.
- Write a program that starts with an array of ten integers. Print all the integers on the terminal. Then print the smallest number in the array.
Concluding remarks
If you haven’t subscribed to our website Computing4All.com and to the YouTube channel where we host our video lectures, please do so to receive notifications on our new articles and videos.
Please do not hesitate to contact us via the comments section below.