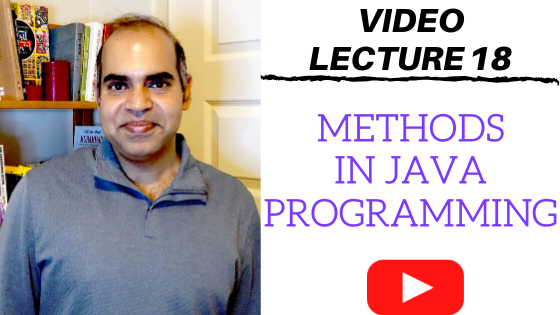
Methods in Java programming language: Video Lecture 18
This article covers details on methods in Java. It explains why we need to use methods in our programs, how to pass data to a method using parameters, and retrieve information from it via a return statement.
A method is a block of code to perform some specific tasks. One method can be called by another method an arbitrary number of times. That means a programmer can write a method when she or he feels that the lines of codes written in the method will be used multiple times.
The use of methods can significantly reduce the length of your code. In many computer languages, methods are known as functions because a method is supposed to code some functionality.
The following video lecture explains methods in Java with examples and tracing.
Contents
Methods in Java
Even though we did not explain what methods in Java are in our previous articles, you are already familiar with one method. That method is the “main
” method that we have been writing in each of our programs throughout our video lecture series on Java programming.
A method has the following format.
ReturnType MethodName (Parameters){
// The body of the method
——-
return …
}
The first line of a method is known as the header. A header generally contains a return type, a method name, and some parameters. Then we write the body of the method. The body contains a standard Java code. It may contain return statements too.
A return type
A return type states what kind of information a method will send to its caller. It can be int
, double
, float
, or any primitive data type. It can be even an object or even an array reference.
Method name
After the return type in the header, we write the method name. The method name cannot be the same as any existing syntax. Also, it has naming rules like — it cannot start with a numerical digit. Uppercase letters are considered different than lower case. It must be one token — which means that there cannot be spaces in the name of the method.
Method parameters
Within parentheses in the header line, we provide parameters. Parameters are inputs to the method. A caller method can send data content to a method using the parameters. We can provide multiple parameters.
The body of a method
We write the body of a method within curly brackets. The body of the code will contain standard Java codes that we are already familiar with. The body can use the parameters as regular variables. It may create new variables, as well. One can return data to the caller by using a return statement.
A return statement
Depending on the logic used in the method, the return statement may appear multiple times in the body, but only one of those return statements will execute. Execution of a return statement will take the program to the caller method, from where this method was called. That is, the execution of a return statement ends the execution of the method where it is situated.
The returned data type must match with the ReturnType
mentioned in the header of the method.
Which method the Java virtual machine executes first?
The Java virtual machine recognizes the main method and always calls it first. Regardless of where we write our methods, before or after the main method, the Java virtual machine will always start the program from the main method.
A simple Java method without any parameter and returns
Let us write our first method, that is not the main method. Let us write a method named myGoodMethod
before the main method.
class MethodTest{
static void myGoodMethod(){
System.out.println("Hi, my name is Shahriar.");
}
public static void main(String[] args){
myGoodMethod();
}
}
Explaining static
In the code above, we start the header line of the method myGoodMethod
with the syntax static
. At this point, it is hard to explain why we are using this syntax static
because we have not yet taught the object-oriented paradigm of Java in our Java lecture series. The explanation that does not include the object-oriented concepts is as follows. From a static method, one can only call other static methods. Since our main method is a static one, and we call the newly created method from our main method, we have to make our new method a static one too.
Return type -void
After writing static
, we need to write a return type. As said earlier, a return type states what kind of information this method will send to its caller. The method, myGoodMethod
, does not return anything. It just prints something. We need to tell Java virtual machine that the method will not return anything. The syntax that states that we will not return anything is void
.
Method name, myGoodMethod
After writing the syntax “void
”, we have to write the method name, which is myGoodMethod
. It could be any other name that does not conflict with any existing Java syntax.
No parameter
In parentheses, we provide nothing. Keeping the parameter list empty indicates that the caller can send nothing through the parameter of this method.
The body of the method
In the body of the method, that is within the curly brackets, we write our code. We wrote a System.out.println
statement to print, “Hi, my name is Shahriar.”
Calling myGoodMethod
from the main method
Now, we can call myGoodMethod
from any method. Therefore, we can call myGoodMethod
from the main method. To call the method myGoodMethod
, we just have to write myGoodMethod
in the main method. We also have to provide the necessary parameters in parentheses. In this case, we do not have any parameters. Therefore, we put an empty set of stating and ending parentheses. Then we put a semicolon like any standard java instructions.
The output of the program
Executing the program will print, “Hi, my name is Shahriar.
” That means, our main method successfully executed the method named myGoodMethod
.
How can we use a parameter in a Java method?
Let us discuss how we can use a parameter. We will change our code a little bit. The goal is to make our method myGoodMethod
suitable for printing any name, not just a specific one. We will send the name as a parameter of the method. The method will print the name that it receives.
Here is the modified code.
class MethodTest{
static void myGoodMethod(String anyName){
System.out.println("Hi, my name is "+anyName+".");
}
public static void main(String[] args){
myGoodMethod("Jane");
myGoodMethod("John");
myGoodMethod("Snow White");
}
}
A parameter in myGoodMethod
When we declare a parameter, we declare it as a variable. We plan to send a name, which is of String
type. Therefore, we state that the parameter is of String
data type in the parameter part of the header. Then we give the parameter a name. The name of the variable is anyName
. Now, the paramter anyName
can be used to send a name from the caller method.
Using the parameter
Whatever content the parameter variable anyName
contains, we want the System.out.println
method to print that. In place of “Shahriar”, we will print the content of the String
variable anyName
. In the System.out.println
, we write “Hi, my name is”, then we add the variable anyName
, and then we add a full-stop symbol.
Now, the method is ready to accept any name from its caller.
Calling with arguments
From the main method, let us call the same method three times, with three different names as the parameter.
In the first call, we provide “Jane” as the argument.
The second call has the argument “John”.
In the third call, we send “Snow White”.
The instances we pass from the caller are sometimes called arguments. That is Jane, john, and Snow White are three arguments we are passing to the method myGoodMethod via three calls.
The parameter anyName
is the variable that captures each of the arguments.
The output of the program
Once we run this program, we should see three lines starting with “Hi, My name is”, but each line will have a different name, Jane, John, and Snow White.
Here is the output of the program.
Hi, my name is Jane.
Hi, my name is John.
Hi, my name is Snow White.
Now, we know how to send a parameter. Let us extend this code a bit and learn how to send two arguments in each call.
Sending two arguments in a method
Sending two or more arguments to a method is not that difficult. We can provide parameters separated by commas.
In our running example, we will use a second paramter to include age of the person whose name is in the first parameter.
Here is the modified class.
class MethodTest1{
static void myGoodMethod(String anyName, int theAge){
System.out.println("Hi, my name is "+anyName+". I am "+theAge);
}
public static void main(String[] args){
myGoodMethod("Jane", 20);
myGoodMethod("John", 19);
myGoodMethod("Snow White", 23);
}
}
In this case, let us say that age is an integer. Therefore, we write int then a variable name theAge
. We separate the two paramters, anyName
and theAge
, by a comma.
Now, we can use the variable theAge
to pass an integer along with the string anyName
.
We change our System.out.println
method to the following one, which includes both the variables, anyName
and theAge
.
System.out.println("Hi, my name is "+anyName+". I am "+theAge);
In the main method, in each of these calls, we have to provide two parameters. The first parameter must be a string, which is inside quotes just like before. There should be a second parameter, which must be an integer because this is how we designed our method named myGoodMethod (String anyName, int theAge)
.
With each name, we provide a second argument in each call, which is an integer. The call myGoodMethod(“Jane”, 20);
indicates that Jane is 20 years old. The next two calls in the main method imply that John is 19 years old, and Snow White is 23 years old.
Once we execute the program, the corresponding age is printed with each name. Here is the output of the program.
Hi, my name is Jane. I am 20
Hi, my name is John. I am 19
Hi, my name is Snow White. I am 23
Another program with parameters and a return value
So far, we have seen how we can pass arguments to a method. We have not yet seen how we can return something from a method to the caller.
We can use the return statement and the return type to return content to the caller.
Let us work on a different problem now.
The problem and the solution
Let i
and j
be two integers. i<=j
. Compute the summation of all the integers starting from i
ending at j
.
That is, if i=3
and j=6
, then find the summation 3+4+5+6
.
For the numbers 3, 4, 5, and 6, the summation will be 18.
We will write a method that will take i
and j
as its parameters. The method will return the summation of all integer numbers between i
and j
. That is, if i is 3 and j is 6, then 18 will be returned by the method.
Here is the program.
class MethodTest2{
static int sumitoj(int i, int j){
int sum=0;
int a;
for (a=i; a<=j; a=a+1){
sum=sum+a;
}
return sum;
}
public static void main(String[] args){
int s;
s=sumitoj(3, 6);
System.out.print("The summation of all integers ");
System.out.println("between 3 and 6 is "+s);
s=sumitoj(10, 20);
System.out.print("The summation of all integers ");
System.out.println("between 10 and 20 is "+s);
s=sumitoj(1, 100);
System.out.print("The summation of all integers ");
System.out.println("between 1 and 100 is "+s);
}
}
Parameters
The name of the method is sumitoj
. sumitoj
has two parameters, i
and j
. It returns an integer because the summation of some integers is an integer. The summation can be considered long too but we will keep the return type an integer with an assumption that the summation will not exceed the range on an integer variable.
Summing the numbers
In the body of the method sumitoj
, we declare an integer variable named sum
. We will go over every integer between i
and j
and put the summation in the variable sum.
sum=0
in the beginning.
We write a for loop. We use a loop variable named a
. The variable “a
” starts from i
, goes over every integer, and ends at j
.
Inside the loop, we add whatever number we see in the variable “a
” with the current sum. Then we remember this summation in the sum variable by assigning the summation to the variable sum
(sum=sum+a;
).
Once the execution comes outside the loop, the variable sum
contains the summation of all the numbers between i
and j
.
Return
We then return the value of sum
. To do that, we write the following statement.
return sum;
sum
is an integer. The return type is also an integer, as we declared. Therefore, the method should work fine.
Calling sumitoj
from the main method
We call the method, sumitoj
, from the main method three times with parameters
- 3 and 6,
- 10 and 20, and
- 1 to 100.
sumitoj(3, 6)
returns 18, as expected. sumitoj(10, 20)
, and sumitoj(1, 100)
return 165 and 5050, respectively. The output of the program reflects the summations. Here is the output of the program.
The summation of all integers between 3 and 6 is 18
The summation of all integers between 10 and 20 is 165
The summation of all integers between 1 and 100 is 5050
The video lecture linked with this article provides a detailed tracing of the program. The video further clarifies how methods execute in a Java program.
Concluding remarks
A programmer generally writes methods for most commonly used functionalities. Instead of writing the same code over and over in the main method, we can call the corresponding method with different arguments.
We will be back with more content soon. To keep in touch, subscribe to our website computing4all.com and to our video channel where we upload our video lectures.
Hope to see you soon in another video. Thank you.