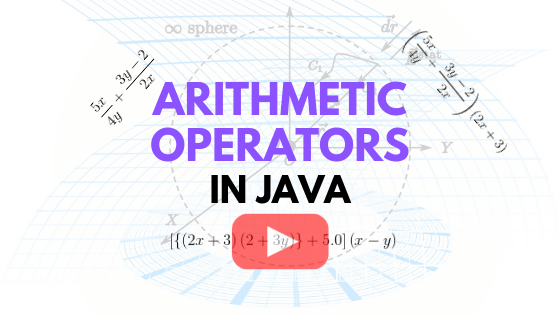
How to Use Arithmetic Operators in Java: Video Lecture 5
Arithmetic operators play a significant role in mathematical operations when using a programming language to solve a problem. The Java programming language has five basic arithmetic operators — addition (+), subtraction (-), multiplication (*), division, and the remainder (%). Programmers apply each of these operators on two operands (that is, on two numbers).
Contents
- 1 The Video: Arithmetic Operators in Java
- 2 Programs Used in the Video
- 3 Exercise
- 4 Additional Resource
- 5 Comment
- 6 Transcription of the Audio of the Video
- 6.1 The Arithmetic Operators in Java
- 6.2 What is the Remainder Operator
- 6.3 Evaluating an Arithmetic Expression
- 6.4 Use Parentheses Instead of Curly or Square Braces in Java Arithmetic Expressions
- 6.5 Multiplication Symbol has to be Explicit in Java Arithmetic Expressions
- 6.6 Variables are Replaced by their Contents when Executed
- 6.7 Coding Program 1
- 6.8 Running the Program
- 6.9 Changing the Code to Get User Input
- 6.10 Coding Program 2: Area of a Circle
- 6.11 The Third Program
- 6.12 Coding Program 3
- 6.13 Conclusion
The Video: Arithmetic Operators in Java
In the following YouTube video, we explain the use of arithmetic operators in Java using three examples. Each of the three examples involves the implementation of a mathematical expression.
Programs Used in the Video
In this section, we provide the codes of the three programs we wrote in the video.
Program 1: Implementing a Mathematical Expression
In the first program, we implement an arithmetic expression containing two variables. The arithmetic expression is:
{(a+b)(2a+3b)}+3a
The video explains, how curly braces and square brackets of mathematical expressions should be handled in Java. It also explains how to handle implicit operators (for example, multiplication symbols that are not present in the equation above.)
The following program codes the arithmetic expression above. Save the code in a file named Prog.java
.
import java.util.Scanner;
class Prog{
public static void main(String[] args){
double a;
double b;
double res;
Scanner sc = new Scanner(System.in);
System.out.println("Enter the 1st number:");
a=sc.nextDouble();
System.out.println("Enter the 2nd number:");
b=sc.nextDouble();
res = ((a+b)*(2*a+3*b))+3*a;
System.out.println(res);
}
}
Program 2: Compute the Area of a Circle
In geometry, computing the area of a circle given a radius, r requires evaluating the following expression: πr2.
We wrote the following program in the video to calculate the area of a circle. Please save the code in a file named CircleArea.java
.
import java.util.Scanner;
class CircleArea{
public static void main(String[] args){
double area;
double r;
Scanner scan = new Scanner (System.in);
System.out.println("What is the radious?");
r=scan.nextDouble();
area = 3.14159 * r * r;
System.out.println("The area is: "+area);
}
}
Program 3: Compute an Arithmetic Expression
Just like the first program, we implement a mathematical expression in the third program. The expression in the third program is slightly more complex than the one in the first program.
We implemented the following arithmetic expression in the third program.

We wrote the following program in the video to code the equation above. Save the program in a file named ProgExt.java
.
import java.util.Scanner;
class ProgExt{
public static void main(String[] args){
double x;
double y;
double result;
Scanner sc = new Scanner(System.in);
System.out.println("Enter value of x: ");
x=sc.nextDouble();
System.out.println("Enter value of y: ");
y=sc.nextDouble();
result = (5*x)/(4*y)+(3*y-2)/(2*x);
System.out.println("The result is: "+result);
}
}
Exercise
Write a program to evaluate each of the following arithmetic expressions.
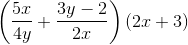


For each of the arithmetic expressions above, using paper and pencil, come up with results for some given values of x and y. For the same x and y, check if your program gives the same result. If the results are different, then you know that either you did it wrong on paper or something is wrong with your code. Please find the issues and fix them. Such fixing of problems is commonly called debugging in the practice of programming.
Additional Resource
The Arithmetic Operators section of the official Java documentation page is a useful reference for the topic we discussed in the video.
Comment
We will cover conditional statements in a future video. Conditional statements help execute different parts of a program under different conditions. To stay in touch, please subscribe to Computing4All.com. Enjoy!
Transcription of the Audio of the Video
Hi, I am Dr. Shahriar Hossain. Today, I am going to explain some items regarding numeric or arithmetic operators in Java. In the previous videos of this lecture series, that Dr. Monika Akbar and I taught, we discussed numeric data types like integers, double, and byte.
We also discussed simple arithmetic operations like addition, subtraction, multiplication, and division. We will describe more on how to use arithmetic operations using Java in this lecture.
The Arithmetic Operators in Java
Java or most standard programming languages have five numeric or arithmetic operators – addition (+), subtraction (-), multiplication (*), division (/), and the remainder (%).
We already worked on addition, subtraction, multiplication, and division in another video. They are basic arithmetic operations and I am sure everyone who is watching this video is familiar with them.
What is the Remainder Operator
What is not common is the remainder operation, which is represented by the percentage symbol (%). In Java, the percentage symbol does not have anything to do with the percentage. After you divide a number by a divisor, you get a quotient and then you have a remainder. The percent symbol or operator in Java will give you the remainder.
For example, 14 remainder 4 is 2, because 4 times three is 12 and then you will have 2 remainder to reach 14. The remainder is computed by the remainder operator.
Anyway, the remainder operations is not hugely common in day-to-day software development. You might need to use the remainder operation if you are working on projects that require implementation of mathematical equations.
Let us talk about how to perform numeric or arithmetic operations in Java.
Evaluating an Arithmetic Expression
You may recall from middle school, how we did simplification of mathematical expressions. We used square brackets, curly braces, and parentheses in arithmetic expressions. The use of curly braces and square brackets is restricted in Java. In arithmetic statements (in Java), we can only use parenthesis.
Use Parentheses Instead of Curly or Square Braces in Java Arithmetic Expressions
For example, in an expression like this one, {(a+b)(2a+3b)}+3a
, we have to replace the opening and ending curly braces with opening and ending parenthesis. Also, we cannot skip any operator when we write arithmetic statements.
Multiplication Symbol has to be Explicit in Java Arithmetic Expressions
On paper, in this expression, ((a+b)(2a+3b))+3a
, we did not provide the multiplication symbols. When using Java, we must explicitly put the multiplication symbols.
Another item, I should mention is that whatever arithmetic statement you write, the result should be kept in a variable, to be able to use it later. For example, if you have a double variable named res, then you can write:
res=((a+b)*(2*a+3*b))+3*a;
Remember from the previous video lectures that the right side of the equals symbol is executed first. The equals symbol is called the assignment operator.
Variables are Replaced by their Contents when Executed
Of course, in place of variables like “a” and “b” Java will put the values in those variables before computing the arithmetic expression you have written in the right side of the assignment operator. After the right side of the assignment is operated, the computed value goes to the variable you have placed in the left side, which is “res”. In this case.
Coding Program 1
Let me quickly code the program.
[Write the code in fast forward mode using equation res=((a+b)*(2*a+3*b))+3*a. Initialize, a to 1.0, and b to 2.0.]
Notice that, I initialized “a” to 1.0 and “b” to 2.0. For these values, I am doing the calculation on paper first. The simplified value is 27. The same way, I did it on paper, the Java Virtual Machine will do it for me using the computer’s processor.
Running the Program
That is if I run the program I have written, the computer will evaluate the arithmetic expression we have written in the right side of the assignment operator of the “res” variable. If I compile and run the program I will be able to check if the program prints 27, as expected.
After compiling and running the program, we see that 27 is printed on the terminal. This demonstrate that whatever we did on paper is actually replicated here using the program. You have written a program that can compute the expression.
Changing the Code to Get User Input
In an ideal case, we will not hard code the initial values like what we did here in our code. We know how to change the code to ask the user for the values of the variables we have.
I will now change the code. I will use a Scanner object that I will use to get the user input for “a” and “b”. After I do that, my code will ask the user for each of the two numbers. The program will take the first number and put it in a. It will take the second number and put it in b.
Let us, save the file, compile it, and run the program. The program is asking for the first number. The user enters 1. It is asking for the second number. The user enters 2. The computer states that the calculated value is 27.
We can run the program again and find the calculated value for any two numbers.
You can write a program to compute the area of a circle, or a rectangle, or a trapezoid, or practically anything as long as you have the arithmetic expression for the problem you are trying solve.
Coding Program 2: Area of a Circle
Let us go over another program. We are writing a program that is able to compute the area of a circle.
Remember from geometry that the area of a circle is πr2.
Pi (π) is constant. That means the value of pi never changes. The value is quite long, but in this program, we will keep only a few digits after the decimal point. We will use π= 3.14159.
Notice that the only variable you have here for the mathematical expression is “r”, which is the radius of the circle. Therefore, we will ask the user to provide the radius. After the user provides the radius, all we have to do is compute π times radius squared.
The radius is stored in the variable “r”. Therefore, we can write “r times r” for radius square. My arithmetic expression becomes, 3.14159*r*r.
We will put the computed value of this expression in a variable named area. Therefore we will print the area.
Let us save the file, compile it, and run the code. The user enters a radius of 2.0. The program computes the area and then prints the area of the circle on the terminal.
The user can run the program again and again to get the areas of circles of different radii.
The Third Program
I will go over one more program now. Suppose, you have this expression
[show on paper 5x/4y+(3y-2)/2x].
How can we code this.
The expression in Java code will be:
(5*x)/(4*y)+(3*y-2)/(2*x)
Notice that there are two variables x and y. Therefore we will ask the user for two numbers, x and y. These two numbers will be kept in two variables named x and y. We will make x and y double numbers. We will keep another variable named “result”, which will hold the computed result.
Coding Program 3
Let us do the coding in fast forward mode. Please pause the video if you are coding it. I will provide the code in the website Computing4All.com too. However, I recommend that you type it yourself. I feel that when you type it yourself, you build a foundational understanding of the program.
Let us save the file, compile it, and run it.
The program asks for the first number and the second number. The program computes the expression for the two given numbers, and prints the computed value.
Conclusion
We will provide exercises regarding today’s video on our website Computing4All.com. As I said before one cannot learn to ride a bike or to swim by watching videos or by reading books. Similar to that, one cannot become skilled in programming just by watching videos or just by reading books. Please practice, practice, and practice to make sure that you develop a strong programming skill over time.